Add authentication to your SvelteKit application
- The following demonstration is built on SvelteKit 2.0.0.
- The sample project is available in the GitHub repository.
Prerequisites
- A Logto Cloud account or a self-hosted Logto.
- A Logto traditional web application created.
Installation
Install Logto SDK via your favorite package manager:
- npm
- pnpm
- yarn
npm i @logto/sveltekit
pnpm add @logto/sveltekit
yarn add @logto/sveltekit
Integration
Add Logto hook
In your hooks.server.ts
file, add the following code to inject the Logto hook into your server:
import { handleLogto } from '@logto/sveltekit';
export const handle = handleLogto(
{
endpoint: '<your-logto-endpoint>',
appId: '<your-logto-app-id>',
appSecret: '<your-logto-app-secret>',
},
{
encryptionKey: '<a-random-string>',
}
);
Since these information are sensitive, it's recommended to use environment variables:
import { handleLogto } from '@logto/sveltekit';
import { env } from '$env/dynamic/private';
export const handle = handleLogto(
{
endpoint: env.LOGTO_ENDPOINT,
appId: env.LOGTO_APP_ID,
appSecret: env.LOGTO_APP_SECRET,
},
{
encryptionKey: env.LOGTO_COOKIE_ENCRYPTION_KEY,
}
);
If you have multiple hooks, you can use the sequence() helper function to chain them:
import { sequence } from '@sveltejs/kit/hooks';
export const handle = sequence(handleLogto, handleOtherHook);
Now you can access the Logto client in the locals
object. For TypeScript, you can add the type to app.d.ts
:
import type { LogtoClient, UserInfoResponse } from '@logto/sveltekit';
declare global {
namespace App {
interface Locals {
logtoClient: LogtoClient;
user?: UserInfoResponse;
}
}
}
We'll discuss the user
object later.
Implement sign-in and sign-out
In the following code snippets, we assume your app is running on http://localhost:3000/
.
Before we dive into the details, here's a quick overview of the end-user experience. The sign-in process can be simplified as follows:
- Your app invokes the sign-in method.
- The user is redirected to the Logto sign-in page. For native apps, the system browser is opened.
- The user signs in and is redirected back to your app (configured as the redirect URI).
Regarding redirect-based sign-in
- This authentication process follows the OpenID Connect (OIDC) protocol, and Logto enforces strict security measures to protect user sign-in.
- If you have multiple apps, you can use the same identity provider (Logto). Once the user signs in to one app, Logto will automatically complete the sign-in process when the user accesses another app.
To learn more about the rationale and benefits of redirect-based sign-in, see Logto sign-in experience explained.
Configure redirect URIs
Switch to the application details page of Logto Console. Add a redirect URI http://localhost:3000/callback
.
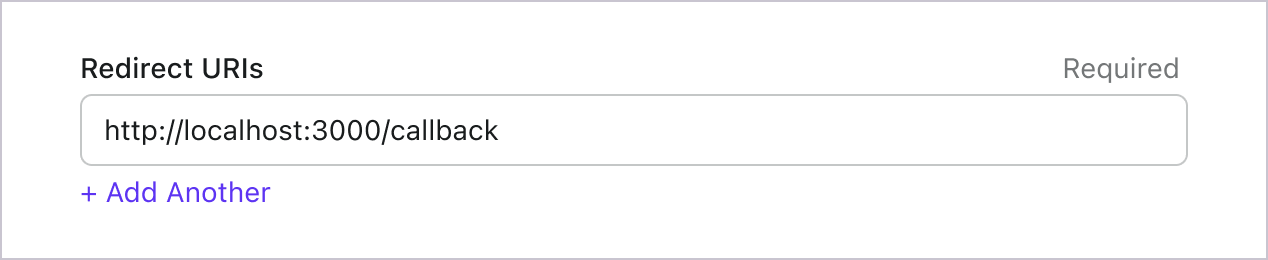
Just like signing in, users should be redirected to Logto for signing out of the shared session. Once finished, it would be great to redirect the user back to your website. For example, add http://localhost:3000/
as the post sign-out redirect URI section.
Then click "Save" to save the changes.
In the page where you want to implement sign-in and sign-out, define the following actions:
import type { Actions } from './$types';
export const actions: Actions = {
signIn: async ({ locals }) => {
await locals.logtoClient.signIn('http://localhost:3000/callback');
},
signOut: async ({ locals }) => {
await locals.logtoClient.signOut('http://localhost:3000/');
},
};
Then use these actions in your Svelte component:
<form method="POST" action="?/{data.user ? 'signOut' : 'signIn'}">
<button type="submit">Sign {data.user ? 'out' : 'in'}</button>
</form>
Checkpoint: Test your application
Now, you can test your application:
- Run your application, you will see the sign-in button.
- Click the sign-in button, the SDK will init the sign-in process and redirect you to the Logto sign-in page.
- After you signed in, you will be redirected back to your application and see the sign-out button.
- Click the sign-out button to clear token storage and sign out.
Get user information
Display user information
To display the user's information, you can inject the locals.user
object into the layout, thus making it available to all pages:
import type { LayoutServerLoad } from './$types';
export const load: LayoutServerLoad = async ({ locals }) => {
return { user: locals.user };
};
In your Svelte component:
<script>
/** @type {import('./$types').PageData} */
export let data;
</script>
{#if data.user}
<ul>
{#each Object.entries(data.user) as [key, value]}
<li>{key}: {value}</li>
{/each}
</ul>
{/if}
Request additional claims
You may find some user information are missing in the returned object from locals.user
. This is because OAuth 2.0 and OpenID Connect (OIDC) are designed to follow the principle of least privilege (PoLP), and Logto is built on top of these standards.
By default, limited claims are returned. If you need more information, you can request additional scopes to access more claims.
A "claim" is an assertion made about a subject; a "scope" is a group of claims. In the current case, a claim is a piece of information about the user.
Here's a non-normative example the scope - claim relationship:
The "sub" claim means "subject", which is the unique identifier of the user (i.e. user ID).
Logto SDK will always request three scopes: openid
, profile
, and offline_access
.
To request additional scopes, you can pass the scopes to the LogtoConfig
object in the handleLogto
function:
import { UserScope, handleLogto } from '@logto/sveltekit';
export const handle = handleLogto({
// ...other options
scopes: [UserScope.email, UserScope.phone], // Add more scopes if needed
});
Then you can access the additional claims in the locals.user
object.
Claims that need network requests
To prevent bloating the user object, some claims require network requests to fetch. For example, the custom_data claim is not included in the user object even if it's requested in the scopes. o access these claims, you can use the client.fetchUserInfo()
method:
By default, the locals.user
object is a convenience of manually calling getIdTokenClaims
method. If you want to use the result of fetchUserInfo
method, you can do so by setting the fetchUserInfo
option to true
for the hook:
import { handleLogto } from '@logto/sveltekit';
export const handle = handleLogto(
{
/* Logto config */
},
{
/* Cookie config */
},
{
fetchUserInfo: true,
}
);
Fetch user information manually
You can use these Logto methods to retrieve user information programmatically:
locals.logtoClient.getIdTokenClaims()
: Get user information by decoding the local ID token. Some claims may not be available.locals.logtoClient.fetchUserInfo()
: Get user information by sending a request to the userinfo endpoint.
It's important to note that the user information claims that can be retrieved depending on the scopes used by the user during signing-in, and considering performance and data size, the ID token may not contain all user claims, some user claims are only available in the userinfo endpoint (see the related list below).
Scopes and claims
Logto uses OIDC scopes and claims conventions to define the scopes and claims for retrieving user information from the ID token and OIDC userinfo endpoint. Both of the "scope" and the "claim" are terms from the OAuth 2.0 and OpenID Connect (OIDC) specifications.
Here's the list of supported scopes and the corresponding claims:
openid
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
sub | string | The unique identifier of the user | No |
profile
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
name | string | The full name of the user | No |
username | string | The username of the user | No |
picture | string | URL of the End-User's profile picture. This URL MUST refer to an image file (for example, a PNG, JPEG, or GIF image file), rather than to a Web page containing an image. Note that this URL SHOULD specifically reference a profile photo of the End-User suitable for displaying when describing the End-User, rather than an arbitrary photo taken by the End-User. | No |
created_at | number | Time the End-User was created. The time is represented as the number of milliseconds since the Unix epoch (1970-01-01T00:00:00Z). | No |
updated_at | number | Time the End-User's information was last updated. The time is represented as the number of milliseconds since the Unix epoch (1970-01-01T00:00:00Z). | No |
Other standard claims include family_name
, given_name
, middle_name
, nickname
, preferred_username
, profile
, website
, gender
, birthdate
, zoneinfo
, and locale
will be also included in the profile
scope without the need for requesting the userinfo endpoint. A difference compared to the claims above is that these claims will only be returned when their values are not empty, while the claims above will return null
if the values are empty.
Unlike the standard claims, the created_at
and updated_at
claims are using milliseconds instead of seconds.
email
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
string | The email address of the user | No | |
email_verified | boolean | Whether the email address has been verified | No |
phone
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
phone_number | string | The phone number of the user | No |
phone_number_verified | boolean | Whether the phone number has been verified | No |
address
Please refer to the OpenID Connect Core 1.0 for the details of the address claim.
custom_data
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
custom_data | object | The custom data of the user | Yes |
identities
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
identities | object | The linked identities of the user | Yes |
sso_identities | array | The linked SSO identities of the user | Yes |
roles
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
roles | string[] | The roles of the user | No |
urn:logto:scope:organizations
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
organizations | string[] | The organization IDs the user belongs to | No |
organization_data | object[] | The organization data the user belongs to | Yes |
urn:logto:scope:organization_roles
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
organization_roles | string[] | The organization roles the user belongs to with the format of <organization_id>:<role_name> | No |
Considering performance and the data size, if "Needs userinfo?" is "Yes", it means the claim will not show up in the ID token, but will be returned in the userinfo endpoint response.
API resources and organizations
We recommend to read 🔐 Role-Based Access Control (RBAC) first to understand the basic concepts of Logto RBAC and how to set up API resources properly.
Configure Logto client
Once you have set up the API resources, you can add them when configuring Logto in your app:
export const handle = handleLogto(
{
// ...other configs
resources: ['https://shopping.your-app.com/api', 'https://store.your-app.com/api'], // Add API resources
}
// ...other configs
);
Each API resource has its own permissions (scopes).
For example, the https://shopping.your-app.com/api
resource has the shopping:read
and shopping:write
permissions, and the https://store.your-app.com/api
resource has the store:read
and store:write
permissions.
To request these permissions, you can add them when configuring Logto in your app:
export const handle = handleLogto(
{
// ...other configs
scopes: ['shopping:read', 'shopping:write', 'store:read', 'store:write'],
resources: ['https://shopping.your-app.com/api', 'https://store.your-app.com/api'],
}
// ...other configs
);
You may notice that scopes are defined separately from API resources. This is because Resource Indicators for OAuth 2.0 specifies the final scopes for the request will be the cartesian product of all the scopes at all the target services.
Thus, in the above case, scopes can be simplified from the definition in Logto, both of the API resources can have read
and write
scopes without the prefix. Then, in the Logto config:
export const handle = handleLogto(
{
// ...other configs
scopes: ['read', 'write'],
resources: ['https://shopping.your-app.com/api', 'https://store.your-app.com/api'],
}
// ...other configs
);
For every API resource, it will request for both read
and write
scopes.
It is fine to request scopes that are not defined in the API resources. For example, you can request the email
scope even if the API resources don't have the email
scope available. Unavailable scopes will be safely ignored.
After the successful sign-in, Logto will issue proper scopes to API resources according to the user's roles.
Fetch access token for the API resource
To fetch the access token for a specific API resource, you can use the getAccessToken
method:
const token = await locals.logtoClient.getAccessToken('https://shopping.your-app.com/api');
This method will return a JWT access token that can be used to access the API resource when the user has related permissions. If the current cached access token has expired, this method will automatically try to use a refresh token to get a new access token.
Fetch organization tokens
If organization is new to you, please read 🏢 Organizations (Multi-tenancy) to get started.
You need to add UserScope.Organizations
scope when configuring the Logto client:
import { handleLogto, UserScope } from '@logto/sveltekit';
export const handle = handleLogto(
{
// ...other configs
scopes: [UserScope.Organizations],
}
// ...other configs
);
Once the user is signed in, you can fetch the organization token for the user:
const token = await locals.logtoClient.getOrganizationToken(organizationId);