Add authentication to your Java Spring Boot application
This guide will show you how to integrate Logto into your Java Spring Boot application.
- You may find the sample code for this guide in our spring-boot-sample github repository.
- No official SDK is required to integrate Logto with your Java Spring Boot application. We will use the Spring Security and Spring Security OAuth2 libraries to handle the OIDC authentication flow with Logto.
Prerequisites
- A Logto Cloud account or a self-hosted Logto.
- Our sample code was created using the Spring Boot securing web starter. Following the instructions to bootstrap a new web application if you don't have one.
- In this guide, we will use the Spring Security and Spring Security OAuth2 libraries to handle OIDC authentication flow with Logto. Please make sure to go through the official documentation to understand the concepts.
Configure your Java Spring Boot application
Adding dependencies
For gradle users, add the following dependencies to your build.gradle
file:
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-security'
implementation 'org.springframework.boot:spring-boot-starter-oauth2-client'
}
For maven users, add the following dependencies to your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
OAuth2 Client Configuration
Register a new Java Spring Boot
application in Logto Console and get the client credential and IdP configurations for your web application.
Add the following configuration to your application.properties
file:
spring.security.oauth2.client.registration.logto.client-name=logto
spring.security.oauth2.client.registration.logto.client-id={{YOUR_CLIENT_ID}}
spring.security.oauth2.client.registration.logto.client-secret={{YOUR_CLIENT_ID}}
spring.security.oauth2.client.registration.logto.redirect-uri={baseUrl}/login/oauth2/code/{registrationId}
spring.security.oauth2.client.registration.logto.authorization-grant-type=authorization_code
spring.security.oauth2.client.registration.logto.scope=openid,profile,offline_access
spring.security.oauth2.client.registration.logto.provider=logto
spring.security.oauth2.client.provider.logto.issuer-uri={{LOGTO_ENDPOINT}}/oidc
spring.security.oauth2.client.provider.logto.authorization-uri={{LOGTO_ENDPOINT}}/oidc/auth
spring.security.oauth2.client.provider.logto.jwk-set-uri={{LOGTO_ENDPOINT}}/oidc/jwks
Implementation
Before we dive into the details, here's a quick overview of the end-user experience. The sign-in process can be simplified as follows:
- Your app invokes the sign-in method.
- The user is redirected to the Logto sign-in page. For native apps, the system browser is opened.
- The user signs in and is redirected back to your app (configured as the redirect URI).
Regarding redirect-based sign-in
- This authentication process follows the OpenID Connect (OIDC) protocol, and Logto enforces strict security measures to protect user sign-in.
- If you have multiple apps, you can use the same identity provider (Logto). Once the user signs in to one app, Logto will automatically complete the sign-in process when the user accesses another app.
To learn more about the rationale and benefits of redirect-based sign-in, see Logto sign-in experience explained.
In order to redirect users back to your application after they sign in, you need to set the redirect URI using the client.registration.logto.redirect-uri
property in the previous step.
Configure redirect URIs
Switch to the application details page of Logto Console. Add a redirect URI http://localhost:3000/callback
.
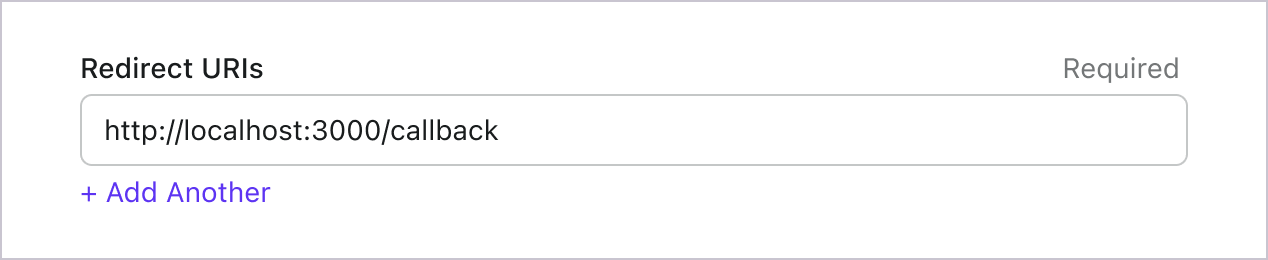
Just like signing in, users should be redirected to Logto for signing out of the shared session. Once finished, it would be great to redirect the user back to your website. For example, add http://localhost:3000/
as the post sign-out redirect URI section.
Then click "Save" to save the changes.
Implement the WebSecurityConfig
Create a new class WebSecurityConfig
in your project
The WebSecurityConfig
class will be used to configure the security settings for your application. It is the key class that will handle the authentication and authorization flow. Please check the Spring Security documentation for more details.
package com.example.securingweb;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
@Configuration
@EnableWebSecurity
public class WebSecurityConfig {
// ...
}
Create a idTokenDecoderFactory
bean
This is required because Logto uses ES384
as the default algorithm, we need to overwrite the default OidcIdTokenDecoderFactory
to use the same algorithm.
import org.springframework.context.annotation.Bean;
import org.springframework.security.oauth2.client.oidc.authentication.OidcIdTokenDecoderFactory;
import org.springframework.security.oauth2.client.registration.ClientRegistration;
import org.springframework.security.oauth2.jose.jws.SignatureAlgorithm;
import org.springframework.security.oauth2.jwt.JwtDecoderFactory;
public class WebSecurityConfig {
// ...
@Bean
public JwtDecoderFactory<ClientRegistration> idTokenDecoderFactory() {
OidcIdTokenDecoderFactory idTokenDecoderFactory = new OidcIdTokenDecoderFactory();
idTokenDecoderFactory.setJwsAlgorithmResolver(clientRegistration -> SignatureAlgorithm.ES384);
return idTokenDecoderFactory;
}
}
Create a LoginSuccessHandler class to handle the login success event
We will redirect the user to the /user
page after a successful login.
package com.example.securingweb;
import java.io.IOException;
import org.springframework.security.core.Authentication;
import org.springframework.security.web.authentication.AuthenticationSuccessHandler;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
public class CustomSuccessHandler implements AuthenticationSuccessHandler {
@Override
public void onAuthenticationSuccess(HttpServletRequest request, HttpServletResponse response,
Authentication authentication) throws IOException, ServletException {
response.sendRedirect("/user");
}
}
Create a LogoutSuccessHandler class to handle the logout success event
Clear the session and redirect the user to the home page.
package com.example.securingweb;
import java.io.IOException;
import org.springframework.security.core.Authentication;
import org.springframework.security.web.authentication.logout.LogoutSuccessHandler;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import jakarta.servlet.http.HttpSession;
public class CustomLogoutHandler implements LogoutSuccessHandler {
@Override
public void onLogoutSuccess(HttpServletRequest request, HttpServletResponse response, Authentication authentication)
throws IOException, ServletException {
HttpSession session = request.getSession();
if (session != null) {
session.invalidate();
}
response.sendRedirect("/home");
}
}
Update the WebSecurityConfig
class with a securityFilterChain
securityFilterChain is a chain of filters that are responsible for processing the incoming requests and responses.
We will configure the securityFilterChain
to allow access to the home page and require authentication for all other requests. Use the CustomSuccessHandler
and CustomLogoutHandler
to handle the login and logout events.
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.web.DefaultSecurityFilterChain;
public class WebSecurityConfig {
// ...
@Bean
public DefaultSecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.authorizeRequests(authorizeRequests ->
authorizeRequests
.antMatchers("/", "/home").permitAll() // Allow access to the home page
.anyRequest().authenticated() // All other requests require authentication
)
.oauth2Login(oauth2Login ->
oauth2Login
.successHandler(new CustomSuccessHandler())
)
.logout(logout ->
logout
.logoutSuccessHandler(new CustomLogoutHandler())
);
return http.build();
}
}
Create a home page
(You may skip this step if you already have a home page in your project)
package com.example.securingweb;
import java.security.Principal;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HomeController {
@GetMapping({ "/", "/home" })
public String home(Principal principal) {
return principal != null ? "redirect:/user" : "home";
}
}
This controller will redirect the user to the user page if the user is authenticated, otherwise, it will show the home page. Add a sign-in link to the home page.
<body>
<h1>Welcome!</h1>
<p><a th:href="@{/oauth2/authorization/logto}">Login with Logto</a></p>
</body>
Create a user page
Create a new controller to handle the user page:
package com.example.securingweb;
import java.security.Principal;
import java.util.Map;
import org.springframework.security.oauth2.client.authentication.OAuth2AuthenticationToken;
import org.springframework.security.oauth2.core.user.OAuth2User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/user")
public class UserController {
@GetMapping
public String user(Model model, Principal principal) {
if (principal instanceof OAuth2AuthenticationToken) {
OAuth2AuthenticationToken token = (OAuth2AuthenticationToken) principal;
OAuth2User oauth2User = token.getPrincipal();
Map<String, Object> attributes = oauth2User.getAttributes();
model.addAttribute("username", attributes.get("username"));
model.addAttribute("email", attributes.get("email"));
model.addAttribute("sub", attributes.get("sub"));
}
return "user";
}
}
Once the user is authenticated, we will retrieve the OAuth2User
data from the authenticated principal object. Please refer OAuth2AuthenticationToken and OAuth2User for more details.
Read the user data and pass it to the user.html
template.
<body>
<h1>User Details</h1>
<div>
<p>
<div><strong>name:</strong> <span th:text="${username}"></span></div>
<div><strong>email:</strong> <span th:text="${email}"></span></div>
<div><strong>id:</strong> <span th:text="${sub}"></span></div>
</p>
</div>
<form th:action="@{/logout}" method="post">
<input type="submit" value="Logout" />
</form>
</body>
Request additional claims
You may find some user information are missing in the returned object from principal (OAuth2AuthenticationToken)
. This is because OAuth 2.0 and OpenID Connect (OIDC) are designed to follow the principle of least privilege (PoLP), and Logto is built on top of these standards.
By default, limited claims are returned. If you need more information, you can request additional scopes to access more claims.
A "claim" is an assertion made about a subject; a "scope" is a group of claims. In the current case, a claim is a piece of information about the user.
Here's a non-normative example the scope - claim relationship:
The "sub" claim means "subject", which is the unique identifier of the user (i.e. user ID).
Logto SDK will always request three scopes: openid
, profile
, and offline_access
.
To retrieve additional user information, you can add extra scopes to the application.properties
file. For example, to request the email
, phone
, and urn:logto:scope:organizations
scope, add the following line to the application.properties
file:
spring.security.oauth2.client.registration.logto.scope=openid,profile,offline_access,email,phone,urn:logto:scope:organizations
Then you can access the additional claims in the OAuth2User
object.
Run and test the application
Run the application and navigate to http://localhost:8080
.
- You will see the home page with a sign-in link.
- Click on the link to sign in with Logto.
- After successful authentication, you will be redirected to the user page with your user details.
- Click on the logout button to sign out. You will be redirected back to the home page.
Scopes and Claims
Logto uses OIDC scopes and claims conventions to define the scopes and claims for retrieving user information from the ID token and OIDC userinfo endpoint. Both of the "scope" and the "claim" are terms from the OAuth 2.0 and OpenID Connect (OIDC) specifications.
In short, when you request a scope, you will get the corresponding claims in the user information. For example, if you request the email
scope, you will get the email
and email_verified
data of the user.
Here's the list of supported scopes and the corresponding claims:
openid
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
sub | string | The unique identifier of the user | No |
profile
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
name | string | The full name of the user | No |
username | string | The username of the user | No |
picture | string | URL of the End-User's profile picture. This URL MUST refer to an image file (for example, a PNG, JPEG, or GIF image file), rather than to a Web page containing an image. Note that this URL SHOULD specifically reference a profile photo of the End-User suitable for displaying when describing the End-User, rather than an arbitrary photo taken by the End-User. | No |
created_at | number | Time the End-User was created. The time is represented as the number of milliseconds since the Unix epoch (1970-01-01T00:00:00Z). | No |
updated_at | number | Time the End-User's information was last updated. The time is represented as the number of milliseconds since the Unix epoch (1970-01-01T00:00:00Z). | No |
Other standard claims include family_name
, given_name
, middle_name
, nickname
, preferred_username
, profile
, website
, gender
, birthdate
, zoneinfo
, and locale
will be also included in the profile
scope without the need for requesting the userinfo endpoint. A difference compared to the claims above is that these claims will only be returned when their values are not empty, while the claims above will return null
if the values are empty.
Unlike the standard claims, the created_at
and updated_at
claims are using milliseconds instead of seconds.
email
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
string | The email address of the user | No | |
email_verified | boolean | Whether the email address has been verified | No |
phone
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
phone_number | string | The phone number of the user | No |
phone_number_verified | boolean | Whether the phone number has been verified | No |
address
Please refer to the OpenID Connect Core 1.0 for the details of the address claim.
custom_data
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
custom_data | object | The custom data of the user | Yes |
identities
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
identities | object | The linked identities of the user | Yes |
sso_identities | array | The linked SSO identities of the user | Yes |
roles
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
roles | string[] | The roles of the user | No |
urn:logto:scope:organizations
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
organizations | string[] | The organization IDs the user belongs to | No |
organization_data | object[] | The organization data the user belongs to | Yes |
urn:logto:scope:organization_roles
Claim name | Type | Description | Needs userinfo? |
---|---|---|---|
organization_roles | string[] | The organization roles the user belongs to with the format of <organization_id>:<role_name> | No |
Considering performance and the data size, if "Needs userinfo?" is "Yes", it means the claim will not show up in the ID token, but will be returned in the userinfo endpoint response.
Add extra scopes and claims at the application.properties
file to request for more user information. For example, to request the urn:logto:scope:organizations
scope, add the following line to the application.properties
file:
spring.security.oauth2.client.registration.logto.scope=openid,profile,offline_access,urn:logto:scope:organizations
User organization claims will be included in the authorization token.