π Interact with Management API
What is Logto Management APIβ
The Logto Management API is a comprehensive set of APIs that gives developers full control over their implementation to suit their product needs and tech stack. It is pre-built, listed in the API resource list in the Logto Console, and cannot be deleted or modified.
Its identifier is in the pattern of https://[tenant-id].logto.app/api
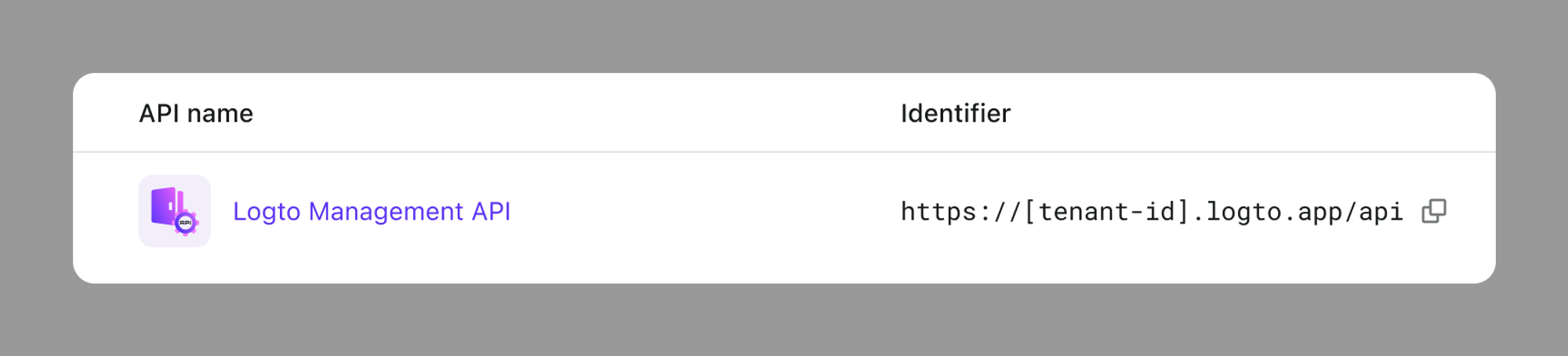
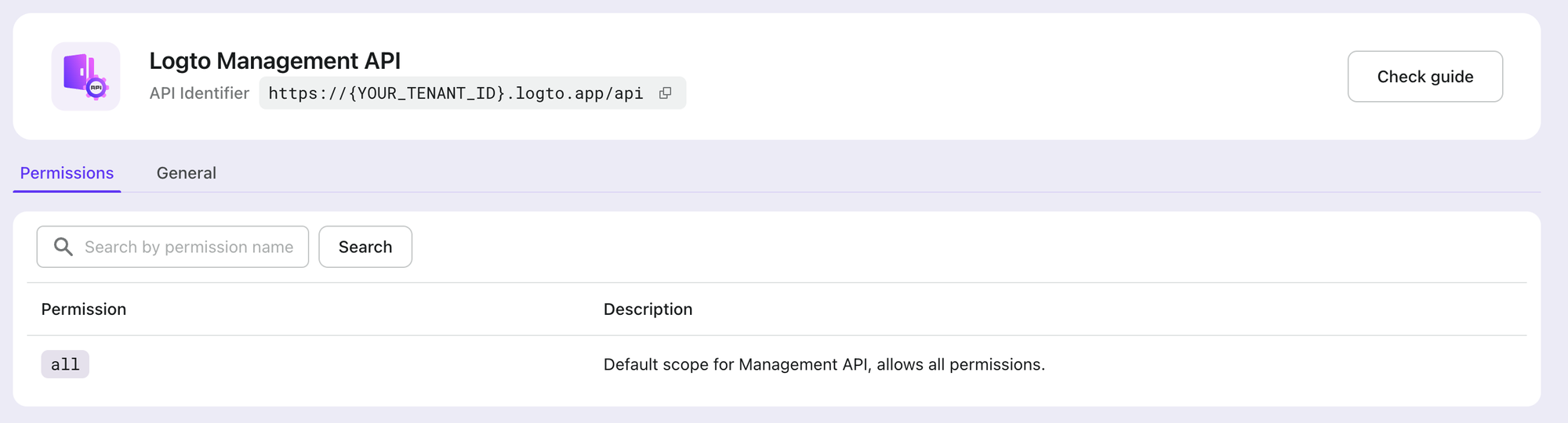
With the Logto Management API, you can access Logto's robust backend services, which are highly scalable and can be utilized in a multitude of scenarios. It goes beyond what's possible with the Admin Console's low-code capabilities.
Some frequently used APIs are listed below:
- User
- Application
- Logs
- Roles
- Resources
- Connectors
To learn more about the APIs that are available, please visit https://openapi.logto.io/.
How to access Logto Management APIβ
Create an M2M appβ
On the "Applications" page, select the M2M app type and start the creation process.
During the M2M app creation process, youβll be directed to a page where you can assign M2M roles to your applications:
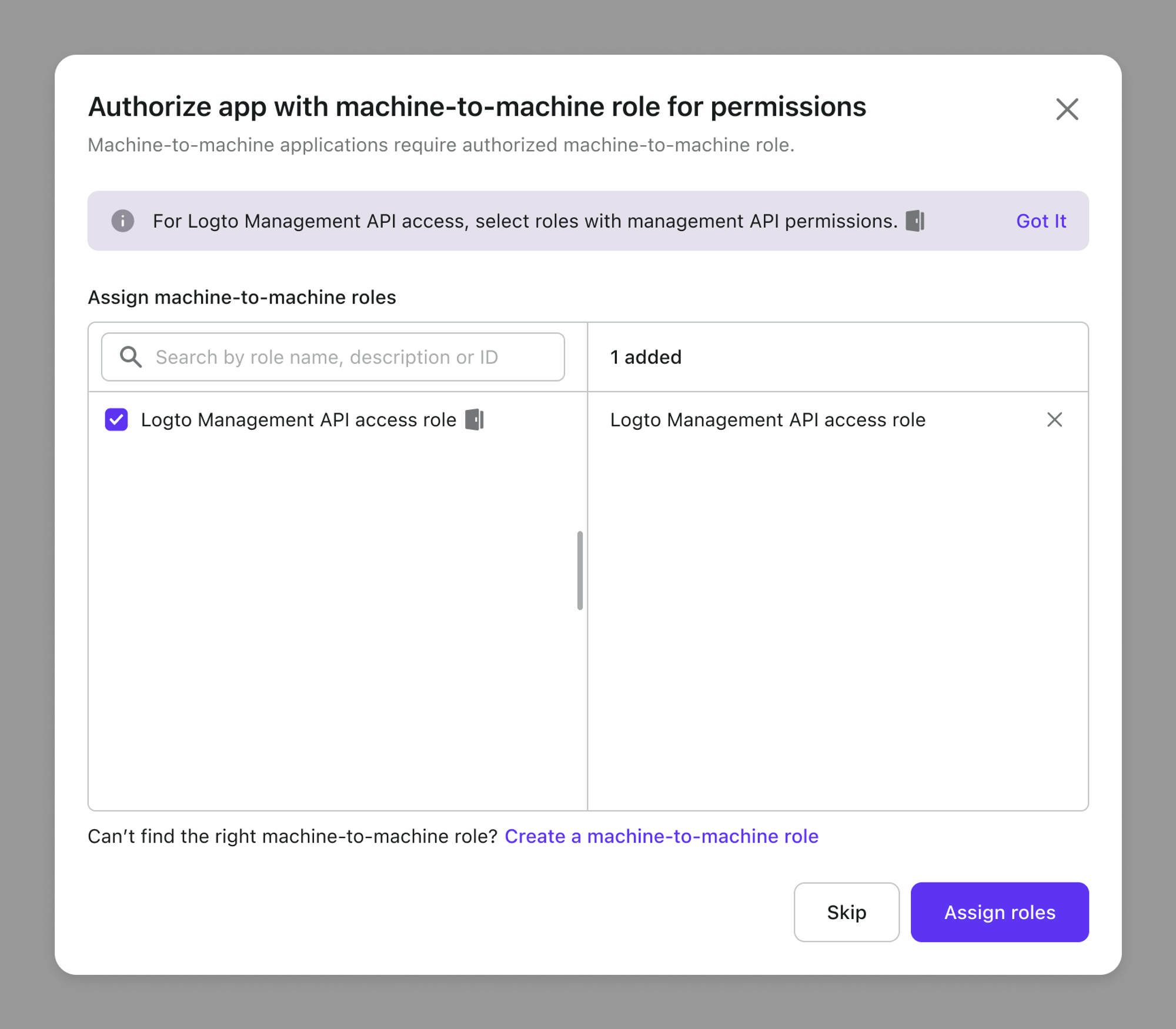
Or you can also assign these roles on the M2M app detail page when you already have an M2M app created:
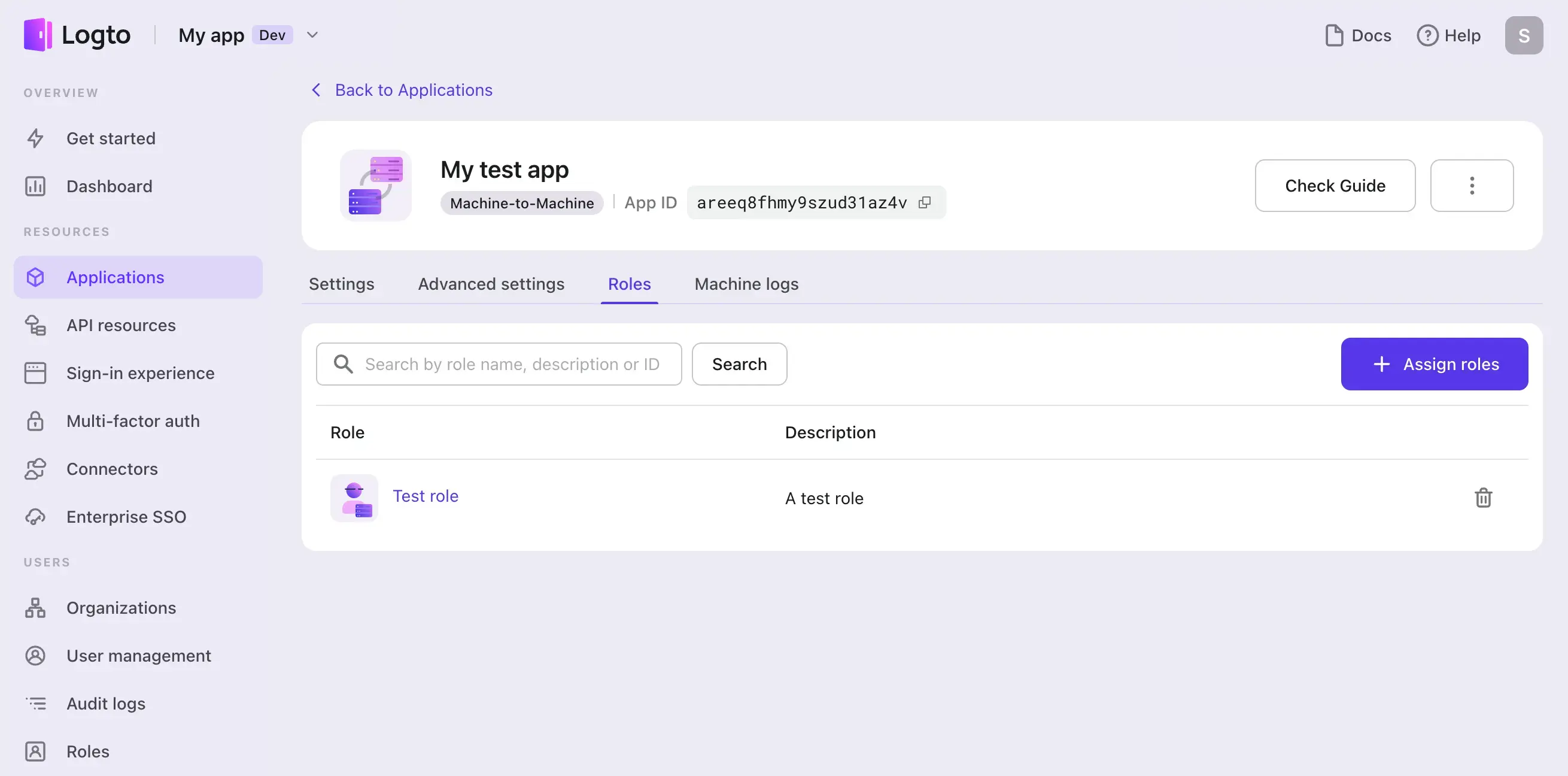
In the role assignment module, you can see all M2M roles are included, and roles indicated by a Logto icon means that these roles include Logto Management API permissions.
Now assign M2M roles include Logto Management API permissions for your M2M app.
Fetch an access tokenβ
Basics about access token requestβ
M2M app makes a POST
request to the token endpoint to fetch an access token by adding the following parameters using the application/x-www-form-urlencoded
format in the HTTP request entity-body:
- grant_type: Must be set to
client_credentials
- resource: The resource you want to access
- scope: The scope of the access request
And you also need to include your M2M app's credentials in the request header for the token endpoint to authenticate your M2M app.
This is achieved by including the app's credentials in the Basic authentication form in the request Authorization
header, where username is the App ID, and password is the App Secret.
You can find the App ID and App Secret from your M2M app's details page:
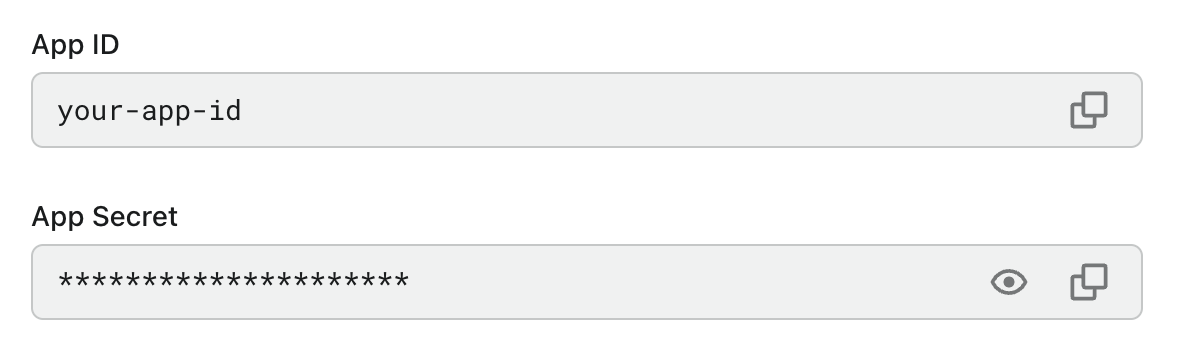
Fetch access token for Logto Management APIβ
Logto provides a built-in βLogto Management APIβ resource, itβs a readonly resource with the all
permission to access Logto Management API, you can see it from your API resource list.
The resource API indicator is in the pattern of https://{your-tenant-id}.logto.app/api
, and this will be your resource value used in the access token request body.
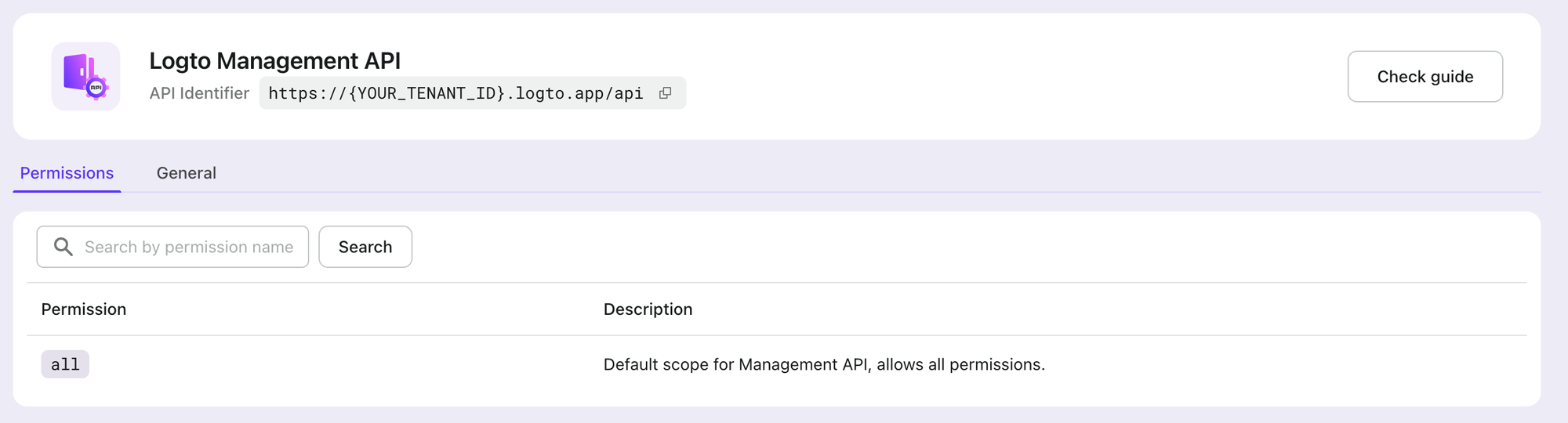
Before accessing Logto Management API, make sure your M2M app has been assigned with M2M roles that include the all
permission from this built-in βLogto Management APIβ resource.
Logto also provides a pre-configured βLogto Management API accessβ M2M role for new created tenants, which the Logto Management API resourceβs all permission has already assigned to. You can use it directly without manually setting permissions. This pre-configured role can also be edited and deleted as needed.
Now, compose all we have and send the request:
- Node.js
- cURL
const logtoEndpoint = 'https://your.logto.endpoint'; // Replace with your Logto endpoint
const tokenEndpoint = `${logtoEndpoint}/oidc/token`;
const applicationId = 'your-application-id';
const applicationSecret = 'your-application-secret';
const tenantId = 'your-tenant-id';
const fetchAccessToken = async () => {
return await fetch(tokenEndpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
Authorization: `Basic ${Buffer.from(`${applicationId}:${applicationSecret}`).toString(
'base64'
)}`,
},
body: new URLSearchParams({
grant_type: 'client_credentials',
resource: `https://${tenantId}.logto.app/api`,
scope: 'all',
}).toString(),
});
};
curl --location \
--request POST 'https://your.logto.endpoint' \
--header 'Authorization: Basic ${your_auth_string}' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=client_credentials' \
--data-urlencode 'resource=https://${tenantId}.logto.app/api' \
--data-urlencode 'scope=all'
Remember to replace the actual values with your own.
For Logto Cloud users: when youβre interacting with Logto Management API, you can not use custom domain, use the default Logto endpoint https://{your_tenant_id}.logto.app/oidc/token
to grant access tokens.
Access token responseβ
A successful access response body would be like:
{
"access_token": "eyJhbG...2g", // Use this token for accessing the Logto Management API
"expires_in": 3600, // Token expiration in seconds
"token_type": "Bearer", // Auth type for your request when using the access token
"scope": "all" // scope `all` for Logto Management API
}
Logto does not currently support the M2M app to represent a user. The sub
in the access token payload will be the App ID.
Access Logto Management API using access tokenβ
You may notice the token response has a token_type
field, which it's fixed to Bearer
.
Thus you should put the access token in the Authorization
field of HTTP headers with the Bearer format (Bearer YOUR_TOKEN
) when you're interacting with your API resource server.
Using the requested access token with the built-in Logto Management API resource https://[your-tenant-id].logto.app/api
to get all applications in Logto:
- Node.js
- cURL
const logtoEndpoint = 'https://your.logto.endpoint'; // Replace with your Logto endpoint
const accessToken = 'eyJhb...2g'; // Access Token
const fetchLogtoApplications = async () => {
return await fetch(`${logtoEndpoint}/api/applications`, {
method: 'GET',
headers: {
Authorization: `Bearer ${accessToken}`,
},
});
};
curl --location \
--request GET 'https://your.logto.endpoint/api/applications' \
--header 'Authorization: Bearer eyJhbG...2g'
Remember to replace the actual values with your own. The value after Bearer
should be the access token (JWT) you received.
Typical scenarios for using Logto Management APIβ
Our developers have implemented many additional features using Logto Management API. We believe that our API is highly scalable and can support a wide range of your needs. Here are a few examples of scenarios that are not possible with the Logto Admin Console but can be achieved through the Logto Management API.
Implement user profile on your ownβ
Logto currently does not provide a pre-built UI solution for user profiles. We recognize that user profiles are closely tied to business and product attributes. While we work on determining the best approach, we suggest using our APIs to create your own solution. For instance, you can utilize our interaction API, profile API, and verification code API to develop a custom solution that meets your needs. Weβve prepared a dedicated page User Profile for tutorials and guides.
Advanced user searchβ
The Logto Admin Console supports basic search and filtering functions. For advanced search options like fuzzy search, exact match, and case sensitivity, check out our Advanced User Search tutorials and guides.
Implement organization management on your ownβ
If youβre using the organization feature to build your multi-tenant app, you might need the Logto Management API for tasks like organization invitations and member management. For your SaaS product, where you have both admins and members in the tenant, the Logto Management API can help you create a custom admin portal tailored to your business needs. Check out this for more detail.
Tips for using Logto Management APIβ
Managing paginated API responsesβ
Some of the API responses may include many results, the results will be paginated. Logto provides 2 kinds of pagination info.
Using link headersβ
A paginated response header will be like:
Link: <https://logto.dev/users?page=1&page_size=20>; rel="first"
The link header provides the URL for the previous, next, first, and last page of results:
- The URL for the previous page is followed by rel="prev".
- The URL for the next page is followed by rel="next".
- The URL for the last page is followed by rel="last".
- The URL for the first page is followed by rel="first".
Using total-number headerβ
In addition to the standard link headers, Logto will also add a Total-Number
header:
Total-Number: 216
That would be very convenient and useful to show page numbers.
Changing page number and page sizeβ
There are 2 optional query parameters:
page
: indicates the page number, starts from 1, the default value is 1.page_size
: indicates the number of items per page, the default value is 20.
Rate limitβ
This is only for Logto Cloud.
To ensure the reliability and security of our services for all users, we employ a general firewall that monitors and manages traffic to our website. While we do not enforce a strict rate limit, we recommend that users limit their activity to approximately 200 requests every 10 seconds to avoid triggering our protective measures.