Enable third-party AI agent access to your MCP server
This guide walks you through integrating Logto with your MCP server using mcp-auth, allowing you to authenticate users and securely retrieve their identity information using the standard OpenID Connect flow.
You'll learn how to:
- Configure Logto as the authorization server for your MCP server.
- Set up a “whoami” tool in your MCP server to return the current user's identity claims.
- Test the flow with a third-party AI agent (MCP client).
After this tutorial, your MCP server will:
- Authenticate users in your Logto tenant.
- Return identity claims (
sub
,username
,name
,email
, etc.) for the "whoami" tool invocation.
Difference between third-party AI agent (MCP client) and your own MCP client
Let's take a look at an example. Imagine you’re a developer running an MCP server to manage email access and automation.
Official email app (Your own MCP client)
- You provide an official email app for users to read and manage their emails.
- How it works: The official email app connects to your MCP server using Logto to authenticate users. When Alice signs in, she automatically gets access to her emails, no extra permission screens needed, since it’s your trusted app.
Third-party AI agent (Third-party MCP client)
- You’re building an ecosystem around your MCP server, so another developer creates “SmartMail AI” (an AI assistant that can summarize emails and schedule meetings automatically) integrating it as a third-party client.
- How it works: SmartMail AI (third-party MCP client) wants to access user emails via your MCP server. When Alice signs in to SmartMail AI using her account:
- She’s shown a consent screen, asking permission for SmartMail AI to read her emails and calendar.
- Alice can allow or deny this access.
- Only the data she consents to is shared with SmartMail AI, and SmartMail AI cannot access any additional data without explicit re-consent.
This access (permission) control ensures user data safety, even though your MCP server manages all the data, third-party apps like SmartMail AI can only access what the user has explicitly allowed. They cannot bypass this process, as it's enforced by your access control implementation in the MCP server.
Summary
Client type | Example | Consent required? | Who controls it? |
---|---|---|---|
Official email app | Your own email application | No | You (the developer) |
Third-party AI agent | SmartMail AI assistant | Yes | Another developer |
If you want to integrate your MCP server with your own AI agent or app, please refer to the Enable auth for your MCP-powered apps with Logto guide.
Prerequisites
- A Logto Cloud (or self-hosted) tenant
- Node.js or Python environment
Understanding the architecture
- MCP server: The server that exposes tools and resources to MCP clients.
- MCP client: A client used to initiate the authentication flow and test the integration. The third-party AI agent will be used as the client in this guide.
- Logto: Serves as the OpenID Connect provider (authorization server) and manages user identities.
A non-normative sequence diagram illustrates the overall flow of the process:
Due to MCP is quickly evolving, the above diagram may not be fully up to date. Please refer to the mcp-auth documentation for the latest information.
Configure third-party AI agent in Logto
To enable the third-party AI agent to access your MCP server, you need to set up a third-party app in Logto. This app will be used to represent the AI agent and obtain the necessary credentials for authentication and authorization.
Allow developers to create third-party apps in Logto
If you are building a marketplace or want to allow developers to create third-party apps in Logto, you can leverage Logto Management API to create third-party apps programmatically. This allows developers to register their applications and obtain the necessary credentials for authentication.
You'll need to host your own service to handle the client registration process. This service will interact with the Logto Management API to create third-party apps on behalf of developers.
Alternatively, you can manually create third-party apps in Logto Console to get familiar with the process.
Manually create a third-party app in Logto
You can manually create a third-party app in Logto Console for testing purposes or ad-hoc integrations. This is useful when you want to quickly test the integration without implementing a full client-registration flow.
-
Sign in to your Logto Console.
-
Go Applications → Create application → Third-party app -> OIDC.
-
Fill in the app name and other required fields, then click Create application.
-
Click Permissions tab, in the User section, click "Add".
-
In the opened dialog -> User data -> select
profile
,email
permissions, then click Save. -
In the third-party app, configure scopes to request
openid profile email
permissions (scopes).Note:
openid
is required for OIDC, andprofile
andemail
are the permissions you added in the previous step. -
Configure the redirect URI of your third-party application accordingly. Remember to update the redirect URI in Logto as well.
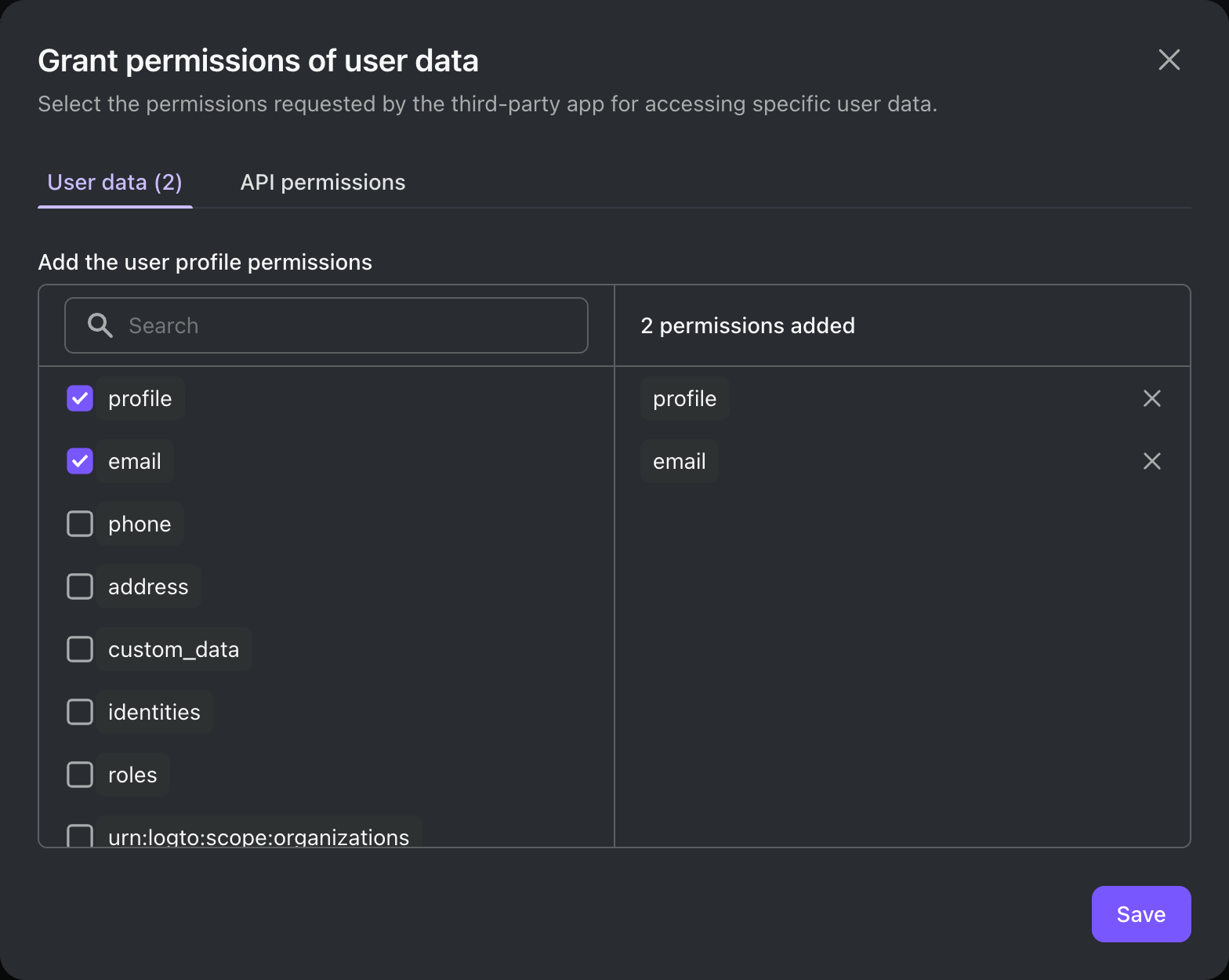
Under the hood, a third-party app is just a standard OAuth 2.0 / OIDC client. This means you (or the third-party developer) can use any OAuth 2.0 / OIDC library or framework to integrate with Logto.
If you're not familiar with OAuth 2.0 or OIDC, you can start by following one of our “Traditional web” quick start guides.
A few things to keep in mind:
- Logto currently requires third-party apps to be “Traditional web” apps. In other words, the app needs a backend server (or backend-for-frontend) to securely store the client secret.
- Most our quick start guides are written for first-party apps, but you can still use them as a reference for third-party app integration.
- The main difference is that third-party apps will show a consent screen, asking users for explicit permission to access their data.
You can find more information in our quick start guides.
Set up the MCP server
Create project and install dependencies
- Python
- Node.js
mkdir mcp-server
cd mcp-server
uv init # Or use your own project structure
uv add "mcp[cli]" starlette uvicorn mcpauth # Or use any preferred package manager
mkdir mcp-server
cd mcp-server
npm init -y
npm install @modelcontextprotocol/sdk express mcp-auth # Or use any preferred package manager
Configure MCP auth with Logto
Remember to replace <your-logto-issuer-endpoint>
with the issuer endpoint you copied earlier.
- Python
- Node.js
In whoami.py
:
from mcpauth import MCPAuth
from mcpauth.config import AuthServerType
from mcpauth.utils import fetch_server_config
auth_issuer = '<your-logto-issuer-endpoint>'
auth_server_config = fetch_server_config(auth_issuer, type=AuthServerType.OIDC)
mcp_auth = MCPAuth(server=auth_server_config)
In whoami.js
:
import { MCPAuth, fetchServerConfig } from 'mcp-auth';
const authIssuer = '<your-logto-issuer-endpoint>';
const mcpAuth = new MCPAuth({
server: await fetchServerConfig(authIssuer, { type: 'oidc' }),
});
Implement token verification
Since we're going to verify the access token and retrieve user info, we need to implement the access token verification as follows:
- Python
- Node.js
import requests
from mcpauth.types import AuthInfo
def verify_access_token(token: str) -> AuthInfo:
endpoint = auth_server_config.metadata.userinfo_endpoint
response = requests.get(
endpoint,
headers={"Authorization": f"Bearer {token}"},
)
response.raise_for_status()
data = response.json()
return AuthInfo(
token=token,
subject=data.get("sub"),
issuer=auth_server_config.metadata.issuer,
claims=data,
)
const verifyToken = async (token) => {
const { userinfoEndpoint, issuer } = mcpAuth.config.server.metadata;
const response = await fetch(userinfoEndpoint, {
headers: { Authorization: `Bearer ${token}` },
});
if (!response.ok) throw new Error('Token verification failed');
const userInfo = await response.json();
return {
token,
issuer,
subject: userInfo.sub,
claims: userInfo,
};
};
Implement the "whoami" tool
Now, let's implement the "whoami" tool that returns the current user's identity claims requesting the userinfo endpoint with the access token sent by the client.
We are using the SSE transport for the example due to the lack of official support for the Streamable HTTP transport in the current version of the SDK. Theoretically, you can use any HTTP-compatible transport.
- Python
- Node.js
from mcp.server.fastmcp import FastMCP
from starlette.applications import Starlette
from starlette.routing import Mount
from starlette.middleware import Middleware
mcp = FastMCP("WhoAmI")
@mcp.tool()
def whoami() -> dict:
"""
Returns the current user's identity information.
"""
return (
mcp_auth.auth_info.claims
if mcp_auth.auth_info
else {"error": "Not authenticated"}
)
bearer_auth = Middleware(mcp_auth.bearer_auth_middleware(verify_access_token))
app = Starlette(
routes=[
mcp_auth.metadata_route(), # Serves OIDC metadata for discovery
Mount('/', app=mcp.sse_app(), middleware=[bearer_auth]),
],
)
Run the server with:
uvicorn whoami:app --host 0.0.0.0 --port 3001
import { McpServer } from '@modelcontextprotocol/sdk/server/mcp.js';
import express from 'express';
// Create MCP server and register the whoami tool
const server = new McpServer({ name: 'WhoAmI', version: '0.0.0' });
server.tool('whoami', ({ authInfo }) => ({
content: [
{ type: 'text', text: JSON.stringify(authInfo?.claims ?? { error: 'Not authenticated' }) },
],
}));
// Express app & MCP Auth middleware
const app = express();
app.use(mcpAuth.delegatedRouter());
app.use(mcpAuth.bearerAuth(verifyToken));
// SSE transport (as in SDK docs)
const transports = {};
app.get('/sse', async (_req, res) => {
const transport = new SSEServerTransport('/messages', res);
transports[transport.sessionId] = transport;
res.on('close', () => delete transports[transport.sessionId]);
await server.connect(transport);
});
app.post('/messages', async (req, res) => {
const sessionId = String(req.query.sessionId);
const transport = transports[sessionId];
if (transport) await transport.handlePostMessage(req, res, req.body);
else res.status(400).send('No transport found for sessionId');
});
app.listen(3001);
Run the server with:
node whoami.js
Test the integration
- Start the MCP server.
- Start the AI agent.
- In the client, invoke the
whoami
tool to retrieve the current user's identity claims. - The client should handle the 401 Unauthorized response and redirect the user to Logto for authentication.
- After successful authentication, the client should receive an access token and use it to make requests to the MCP server.
- The client should be able to retrieve the identity claims from the MCP server using the access token.
- Python
- Node.js
The full MCP server code can be found in the mcp-auth/python repository.
The full MCP server code can be found in the mcp-auth/js repository.