Logto is an Auth0 alternative designed for modern apps and SaaS products. It offers both Cloud and Open-source services to help you quickly launch your identity and management (IAM) system. Enjoy authentication, authorization, and multi-tenant management all in one.
We recommend starting with a free development tenant on Logto Cloud. This allows you to explore all the features easily.
In this article, we will go through the steps to quickly build the Microsoft Entra ID SAML enterprise SSO sign-in experience (user authentication) with Expo (React Native) and Logto.
Prerequisites
- A running Logto instance. Check out the introduction page to get started.
- Basic knowledge of Expo (React Native).
- A usable Microsoft Entra ID SAML enterprise SSO account.
Create an application in Logtoβ
Logto is based on OpenID Connect (OIDC) authentication and OAuth 2.0 authorization. It supports federated identity management across multiple applications, commonly called Single Sign-On (SSO).
To create your Native app application, simply follow these steps:
- Open the Logto Console. In the "Get started" section, click the "View all" link to open the application frameworks list. Alternatively, you can navigate to Logto Console > Applications, and click the "Create application" button.
- In the opening modal, click the "Native app" section or filter all the available "Native app" frameworks using the quick filter checkboxes on the left. Click the "Expo" framework card to start creating your application.
- Enter the application name, e.g., "Bookstore," and click "Create application".
π Ta-da! You just created your first application in Logto. You'll see a congrats page which includes a detailed integration guide. Follow the guide to see what the experience will be in your application.
Integrate Expo with Logtoβ
- The following demonstration is built on Expo ~50.0.6.
- The sample project is available on our SDK repository.
Installationβ
Install Logto SDK and peer dependencies via your favorite package manager:
- npm
- Yarn
- pnpm
npm i @logto/rn
npm i expo-crypto expo-secure-store expo-web-browser @react-native-async-storage/async-storage
yarn add @logto/rn
yarn add expo-crypto expo-secure-store expo-web-browser @react-native-async-storage/async-storage
pnpm add @logto/rn
pnpm add expo-crypto expo-secure-store expo-web-browser @react-native-async-storage/async-storage
The @logto/rn
package is the SDK for Logto. The remaining packages are its peer dependencies. They couldn't be listed as direct dependencies because the Expo CLI requires that all dependencies for native modules be installed directly within the root project's package.json
.
If you're installing this in a bare React Native app, you should also follow these additional installation instructions.
Init Logto providerβ
Import and use LogtoProvider
to provide a Logto context:
import { LogtoProvider, LogtoConfig } from '@logto/rn';
const config: LogtoConfig = {
endpoint: '<your-logto-endpoint>',
appId: '<your-application-id>',
};
const App = () => (
<LogtoProvider config={config}>
<YourAppContent />
</LogtoProvider>
);
Implement sign-in and sign-outβ
Before we dive into the details, here's a quick overview of the end-user experience. The sign-in process can be simplified as follows:
- Your app invokes the sign-in method.
- The user is redirected to the Logto sign-in page. For native apps, the system browser is opened.
- The user signs in and is redirected back to your app (configured as the redirect URI).
Regarding redirect-based sign-inβ
- This authentication process follows the OpenID Connect (OIDC) protocol, and Logto enforces strict security measures to protect user sign-in.
- If you have multiple apps, you can use the same identity provider (Logto). Once the user signs in to one app, Logto will automatically complete the sign-in process when the user accesses another app.
To learn more about the rationale and benefits of redirect-based sign-in, see Logto sign-in experience explained.
Switch to the application details page of Logto Console. Add a native redirect URI (for example, io.logto://callback
), then click "Save".
-
For iOS, the redirect URI scheme does not really matter since the
ASWebAuthenticationSession
class will listen to the redirect URI regardless of if it's registered. -
For Android, the redirect URI scheme must be filled in Expo's
app.json
file, for example:app.json{
"expo": {
"scheme": "io.logto"
}
}
Now back to your app, you can use useLogto
hook to sign in and sign out:
import { useLogto } from '@logto/rn';
import { Button } from 'react-native';
const Content = () => {
const { signIn, signOut, isAuthenticated } = useLogto();
return (
<div>
{isAuthenticated ? (
<Button title="Sign out" onPress={async () => signOut()} />
) : (
// Replace the redirect URI with your own
<Button title="Sign in" onPress={async () => signIn('io.logto://callback')} />
)}
</div>
);
};
Checkpoint: Test your applicationβ
Now, you can test your application:
- Run your application, you will see the sign-in button.
- Click the sign-in button, the SDK will init the sign-in process and redirect you to the Logto sign-in page.
- After you signed in, you will be redirected back to your application and see the sign-out button.
- Click the sign-out button to clear token storage and sign out.
Add Microsoft Entra ID SAML enterprise SSO connectorβ
To simplify access management and gain enterprise-level safeguards for your big clients, connect with Expo as a federated identity provider. The Logto enterprise SSO connector helps you establish this connection in minutes by allowing several parameter inputs.
To add an enterprise SSO connector, simply follow these steps:
- Navigate to Logto console > Enterprise SSO.
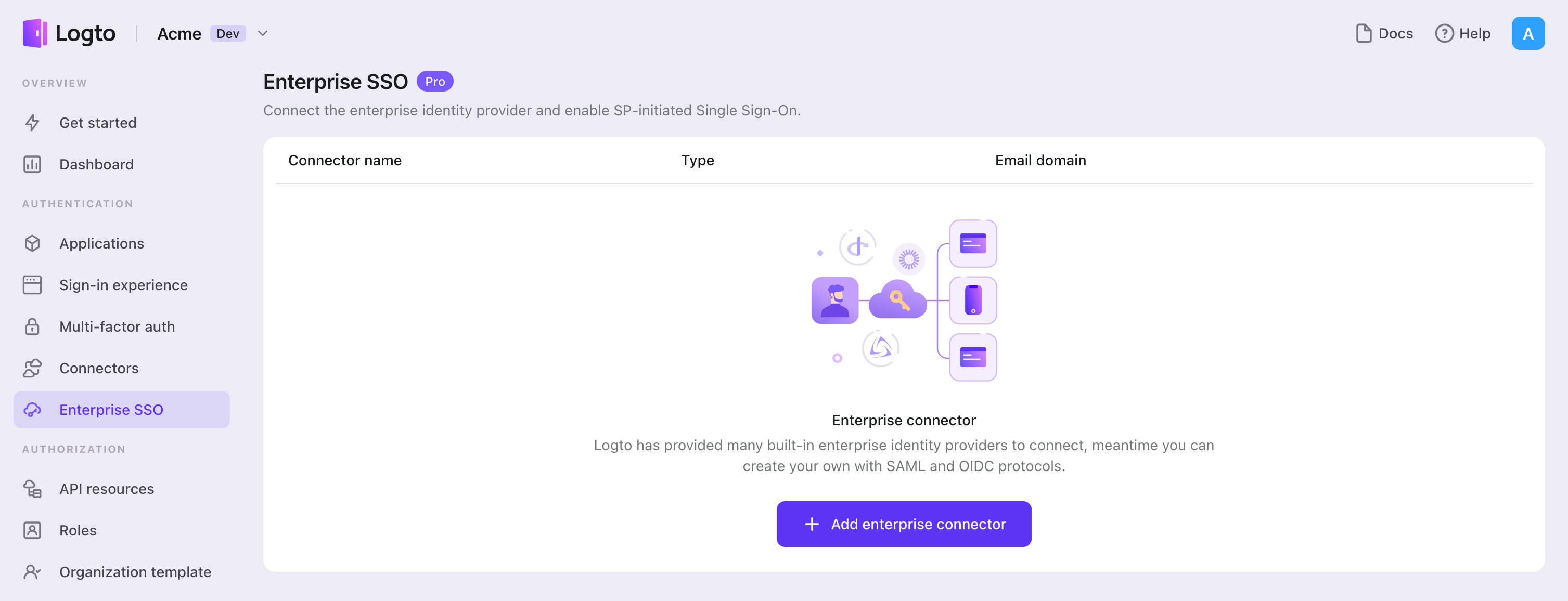
- Click "Add enterprise connector" button and choose your SSO provider type. Choose from prebuilt connectors for Microsoft Entra ID (Azure AD), Google Workspace, and Okta, or create a custom SSO connection using the standard OpenID Connect (OIDC) or SAML protocol.
- Provide a unique name (e.g., SSO sign-in for Acme Company).
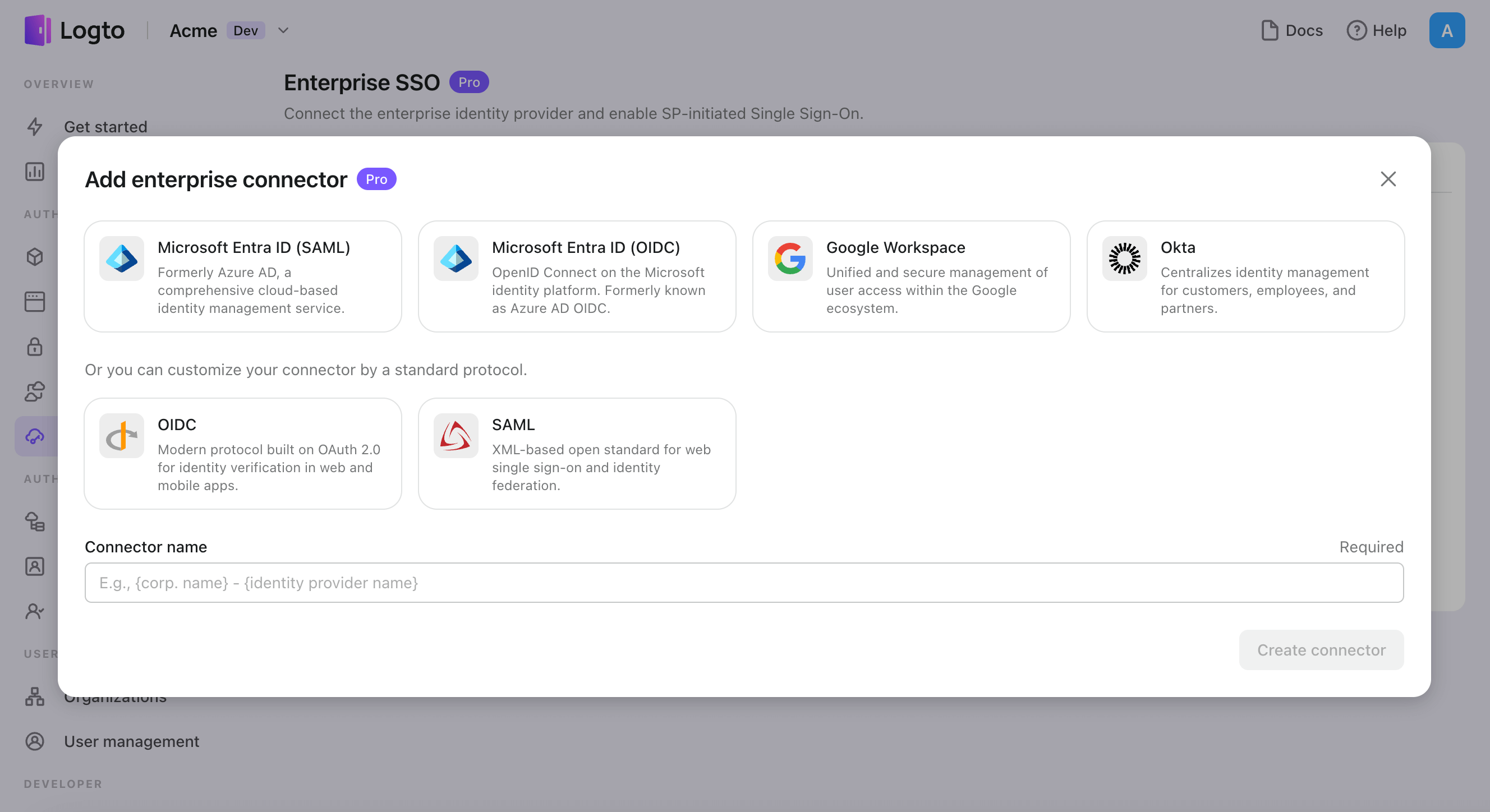
- Configure the connection with your IdP in the "Connection" tab. Check the guides above for each connector types.
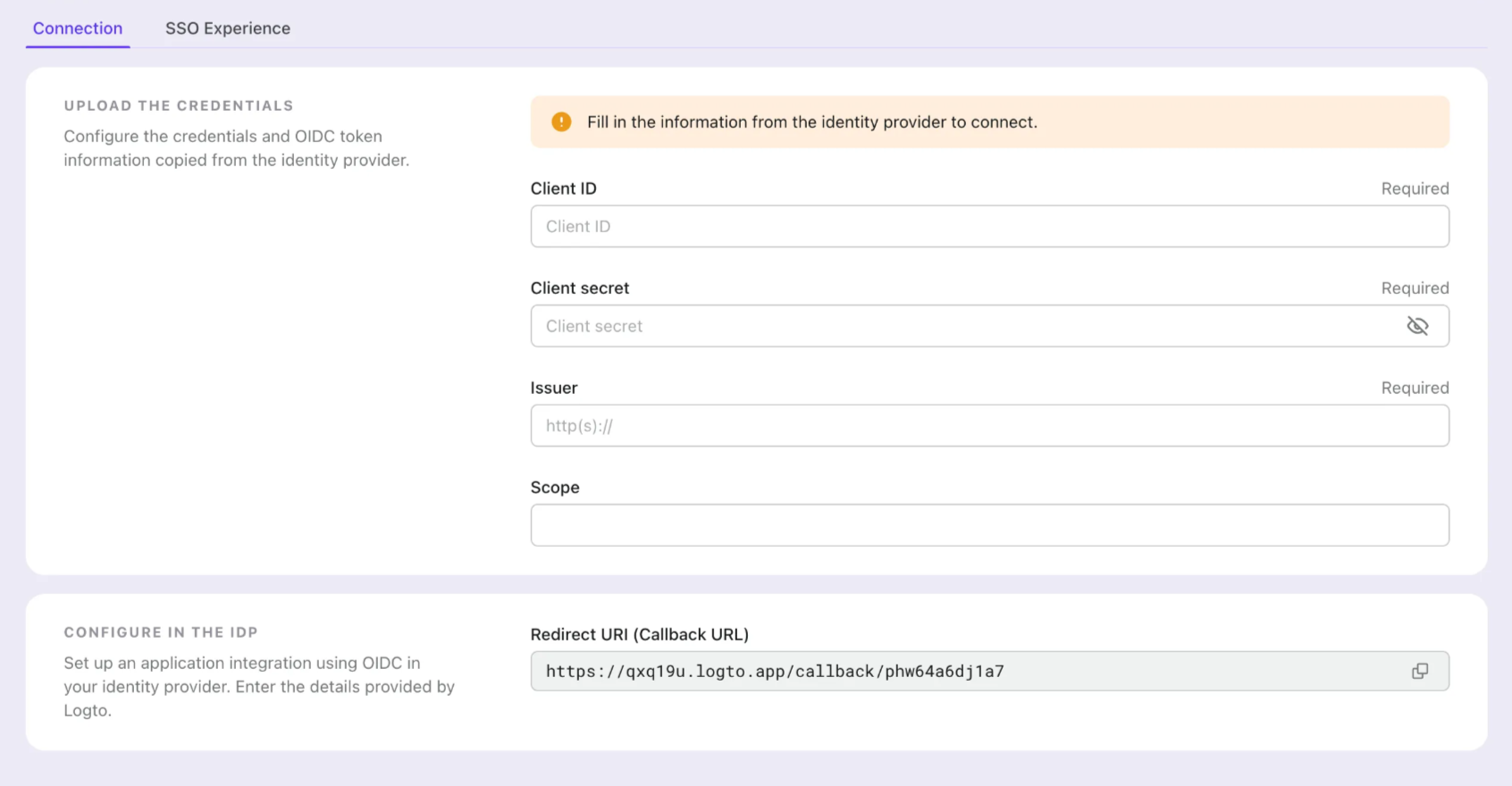
- Customize the SSO experience and enterpriseβs email domain in the "Experience" tab. Users sign in with the SSO-enabled email domain will be redirected to SSO authentication.
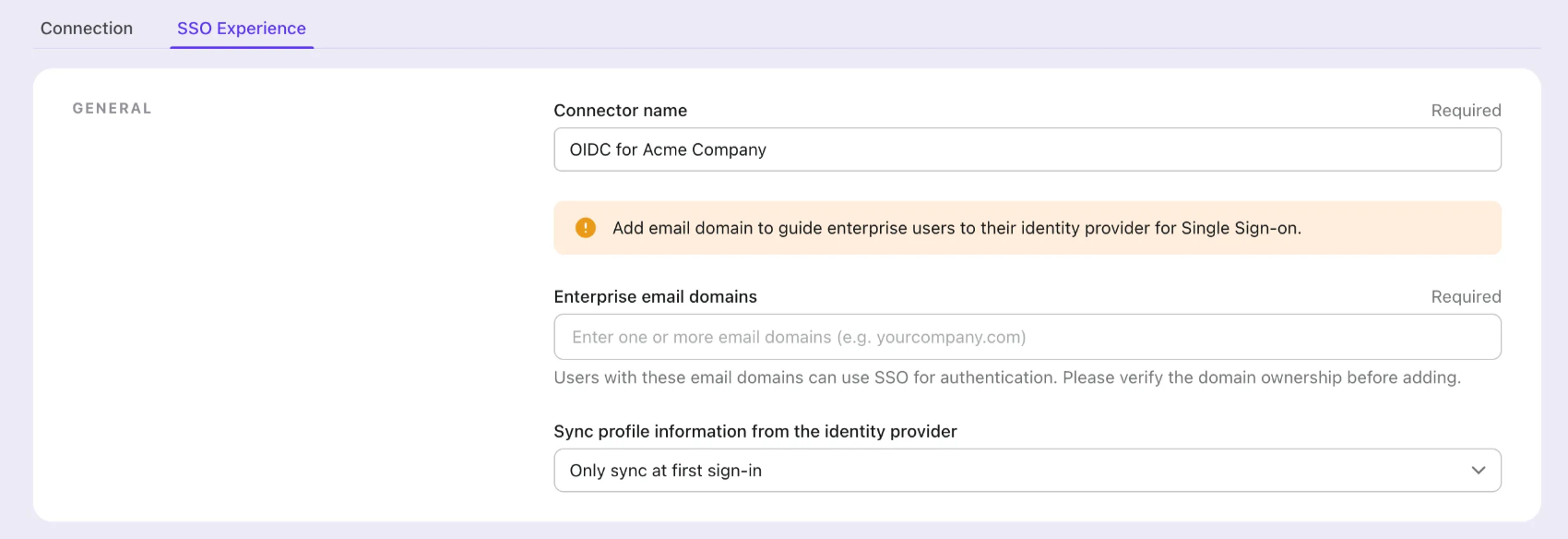
- Save changes.
Set up Azure AD SSO applicationβ
Step 1: Create an Azure AD SSO applicationβ
Initiate the Azure AD SSO integration by creating an SSO application on the Azure AD side.
- Go to the Azure portal and sign in as an administrator.
- Select
Microsoft Entra ID
service. - Navigate to the
Enterprise applications
using the side menu. ClickNew application
, and selectCreate your own application
.
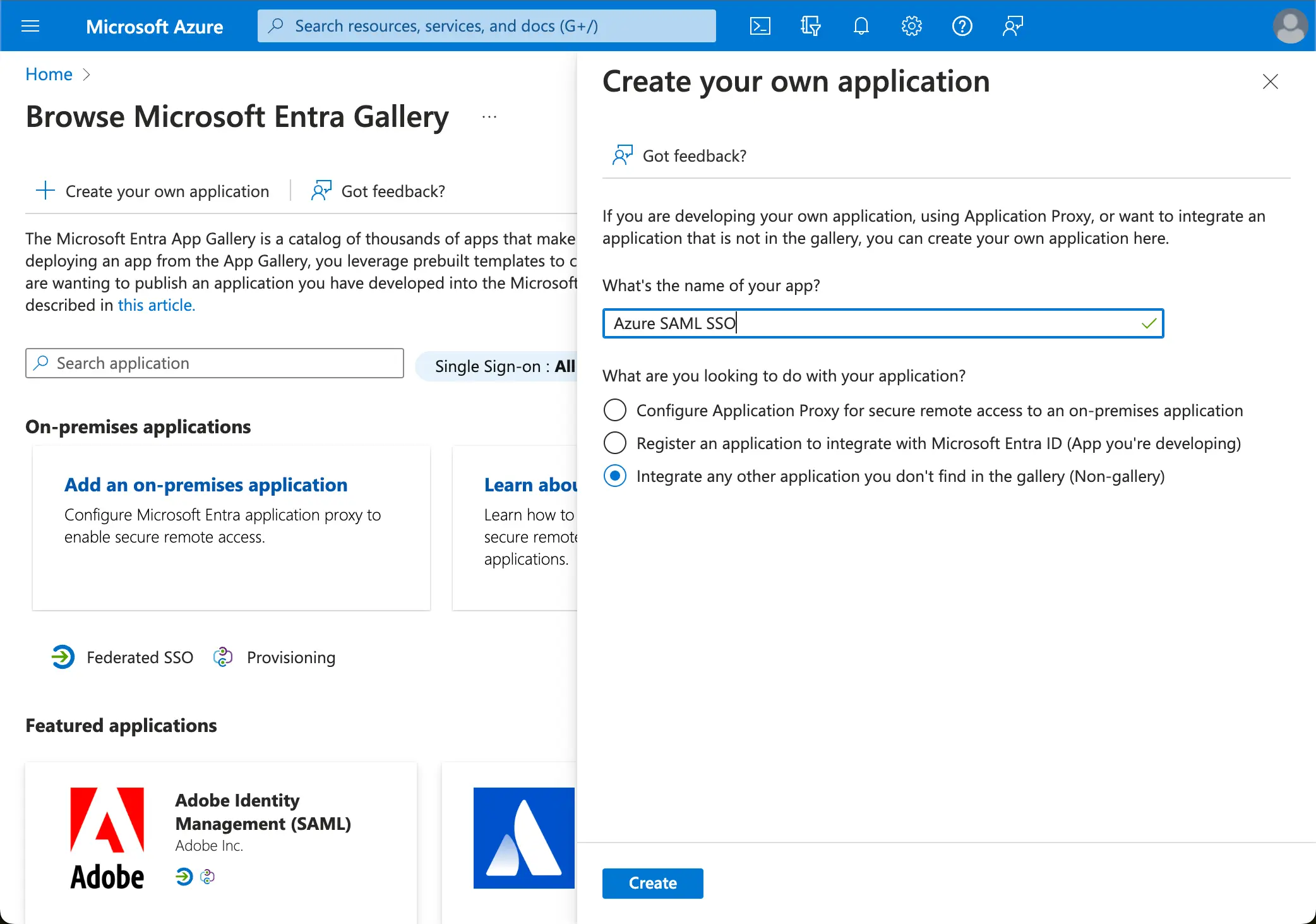
- Enter the application name and select
Integrate any other application you don't find in the gallery (Non-gallery)
. - Select
Setup single sign-on
>SAML
.
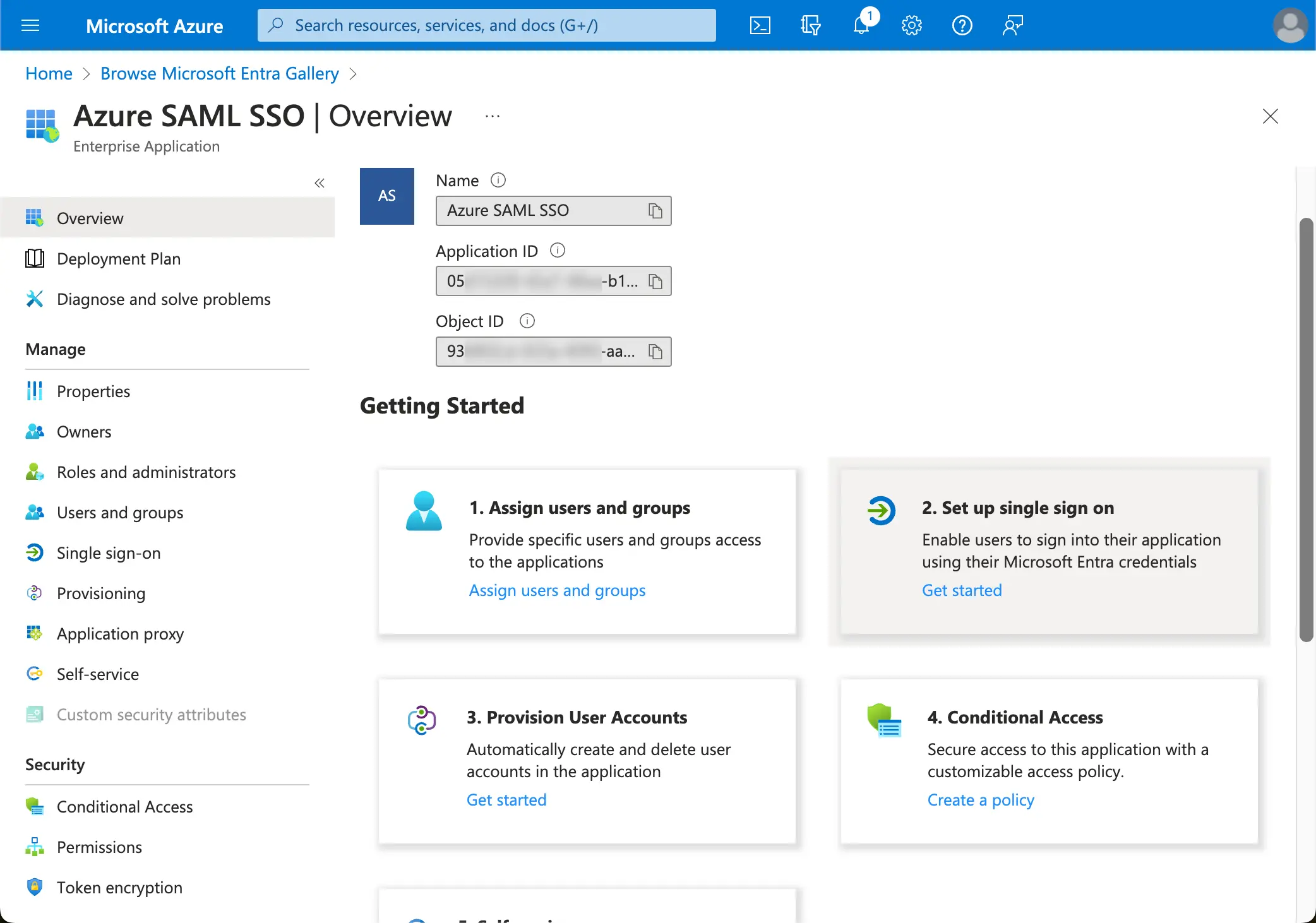
- Follow the instructions, as the first step, you will need to fill in the basic SAML configuration using the following information provided by Logto.
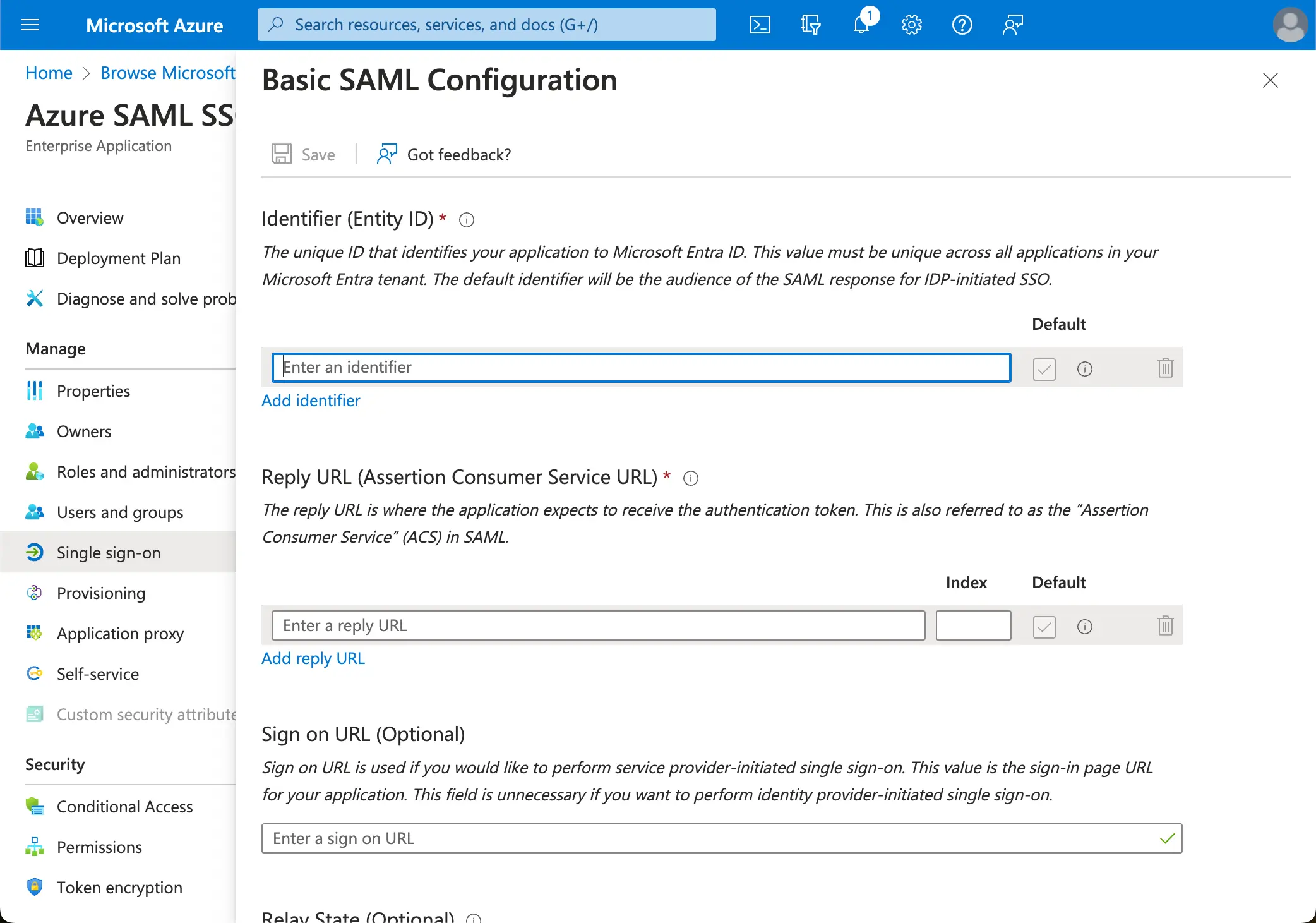
- Audience URI(SP Entity ID): It represents as a globally unique identifier for your Logto service, functioning as the EntityId for SP during authentication requests to the IdP. This identifier is pivotal for the secure exchange of SAML assertions and other authentication-related data between the IdP and Logto.
- ACS URL: The Assertion Consumer Service (ACS) URL is the location where the SAML assertion is sent with a POST request. This URL is used by the IdP to send the SAML assertion to Logto. It acts as a callback URL where Logto expects to receive and consume the SAML response containing the user's identity information.
Click Save
to continue.
Step 2: Configure SAML SSO at Logtoβ
To make the SAML SSO integration work, you will need to provide the IdP metadata back to Logto. Let's switch back to the Logto side, and navigate to the Connection
tab of your Azure AD SSO connector.
Logto provides three different ways to configure the IdP metadata. The easiest way is by providing the metadata URL
of the Azure AD SSO application.
Copy the App Federation Metadata Url
from your Azure AD SSO application's SAML Certificates section
and paste it into the Metadata URL
field in Logto.
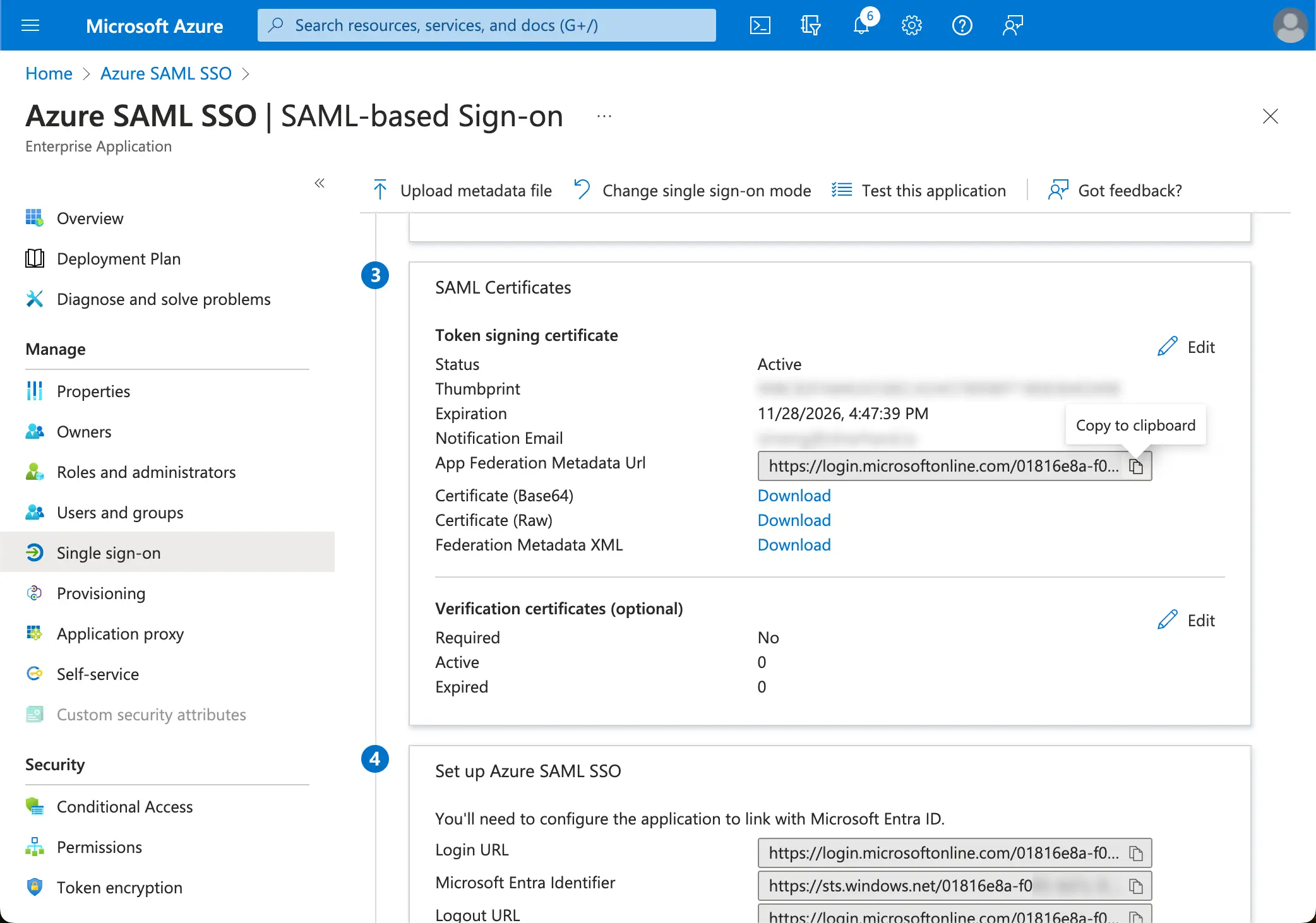
Logto will fetch the metadata from the URL and configure the SAML SSO integration automatically.
Step 3: Configure user attributes mappingβ
Logto provides a flexible way to map the user attributes returned from IdP to the user attributes in Logto. Logto will sync the following user attributes from IdP by default:
- id: The unique identifier of the user. Logto will read the
nameID
claim from the SAML response as the user SSO identity id. - email: The email address of the user. Logto will read the
email
claim from the SAML response as the user primary email by default. - name: The name of the user.
You may manage the user attributes mapping logic either on the Azure AD side or Logto side.
-
Map the AzureAD user attributes to Logto user attributes on Logto side.
Visit the
Attributes & Claims
section of your Azure AD SSO application.Copy the following attribute names (with namespace prefix) and paste them into the corresponding fields in Logto.
http://schemas.xmlsoap.org/ws/2005/05/identity/claims/emailaddress
http://schemas.xmlsoap.org/ws/2005/05/identity/claims/name
(Recommendation: update this attribute value map touser.displayname
for better user experience)a
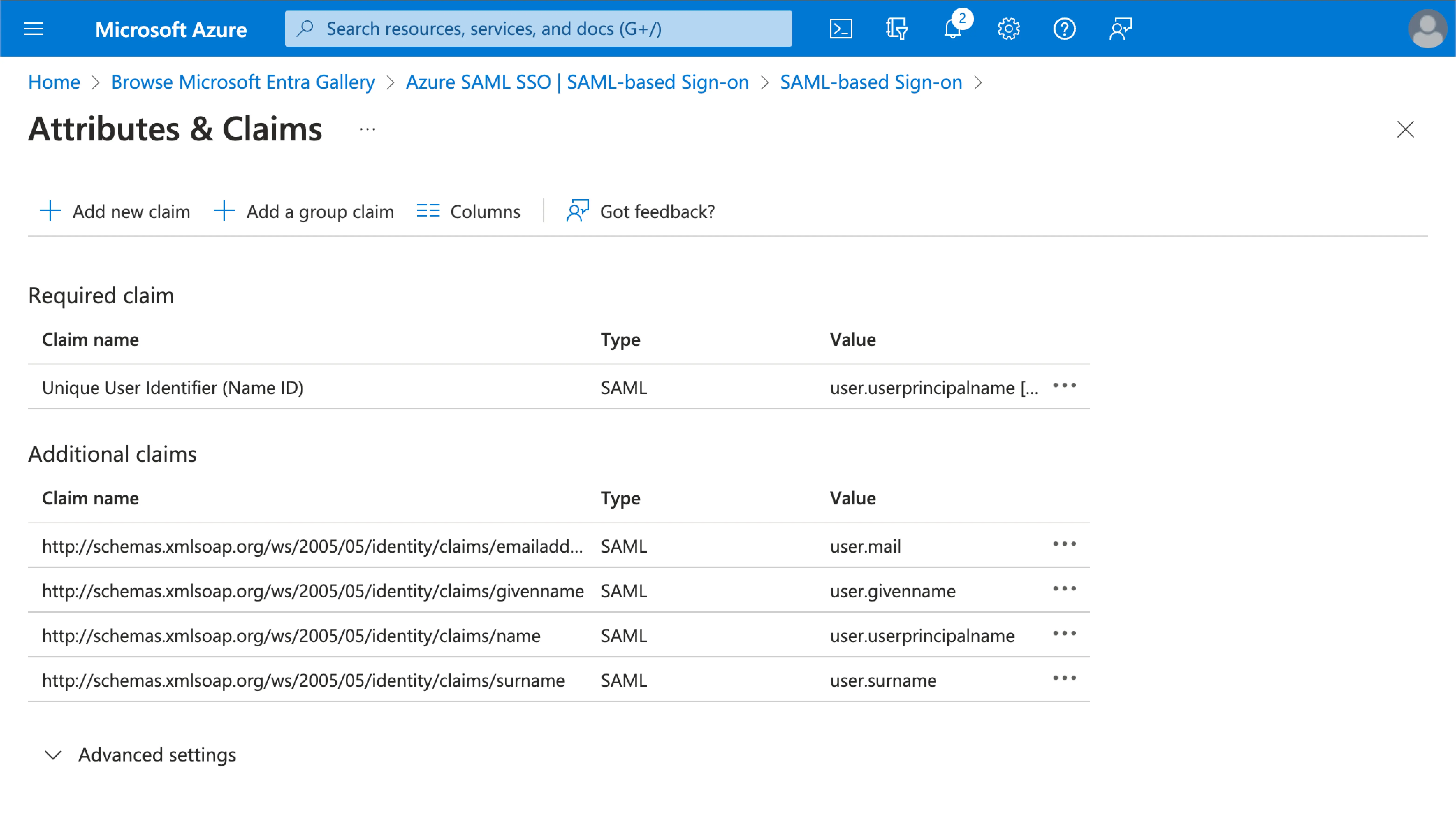
-
Map the AzureAD user attributes to Logto user attributes at the AzureAD side.
Visit the
Attributes & Claims
section of your Azure AD SSO application.Click on
Edit
, and update theAdditional claims
fields based on the Logto user attributes settings:- update the claim name value based on the Logto user attributes settings.
- remove the namespace prefix.
- click
Save
to continue.
Should end up with the following settings:
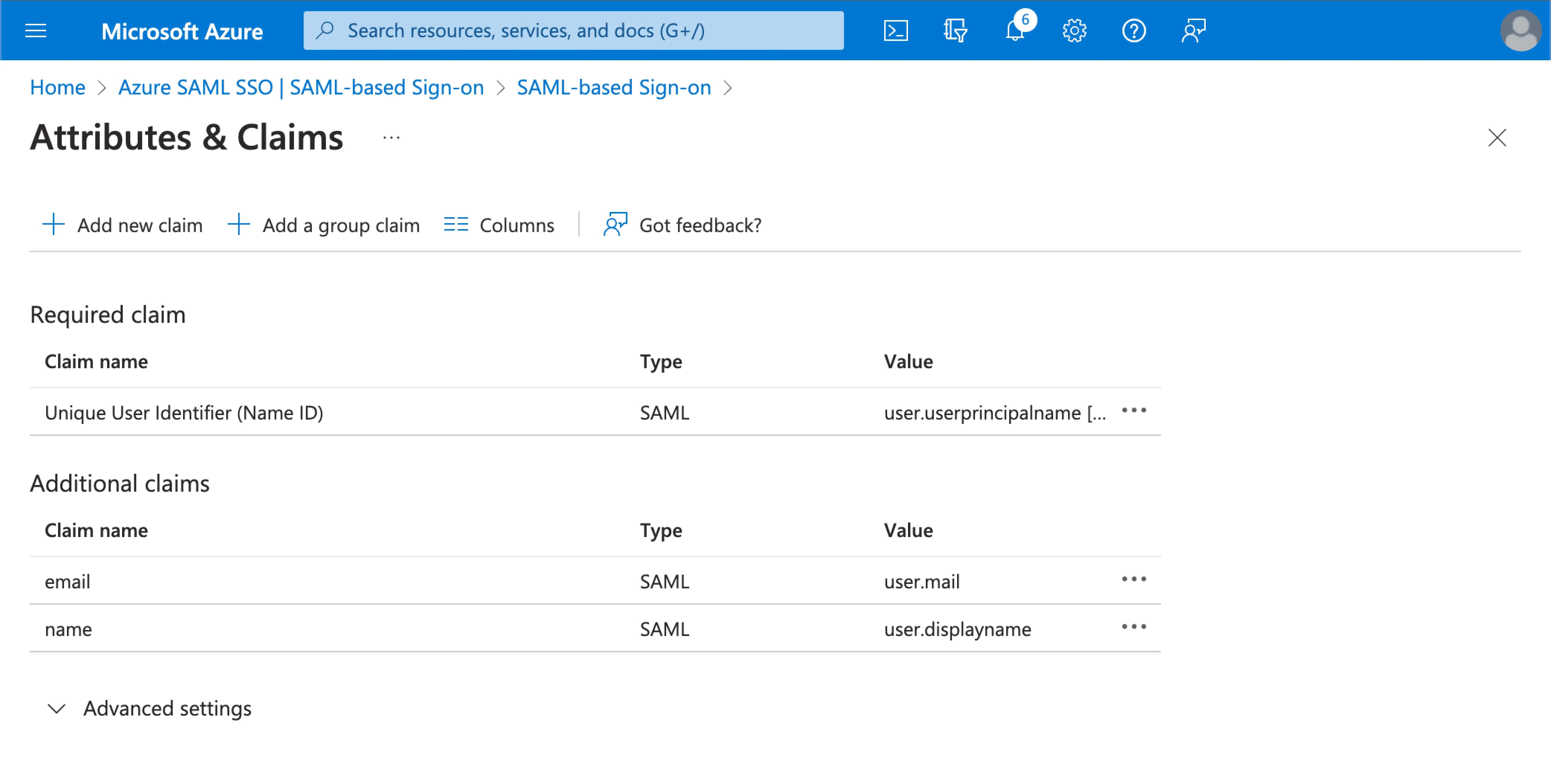
You may also specify additional user attributes on the Azure AD side. Logto will keep a record of the original user attributes returned from IdP under the user's sso_identity
field.
Step 4: Assign users to the Azure AD SSO applicationβ
Visit the Users and groups
section of your Azure AD SSO application. Click on Add user/group
to assign users to the Azure AD SSO application. Only users assigned to your Azure AD SSO application will be able to authenticate through the Azure AD SSO connector.
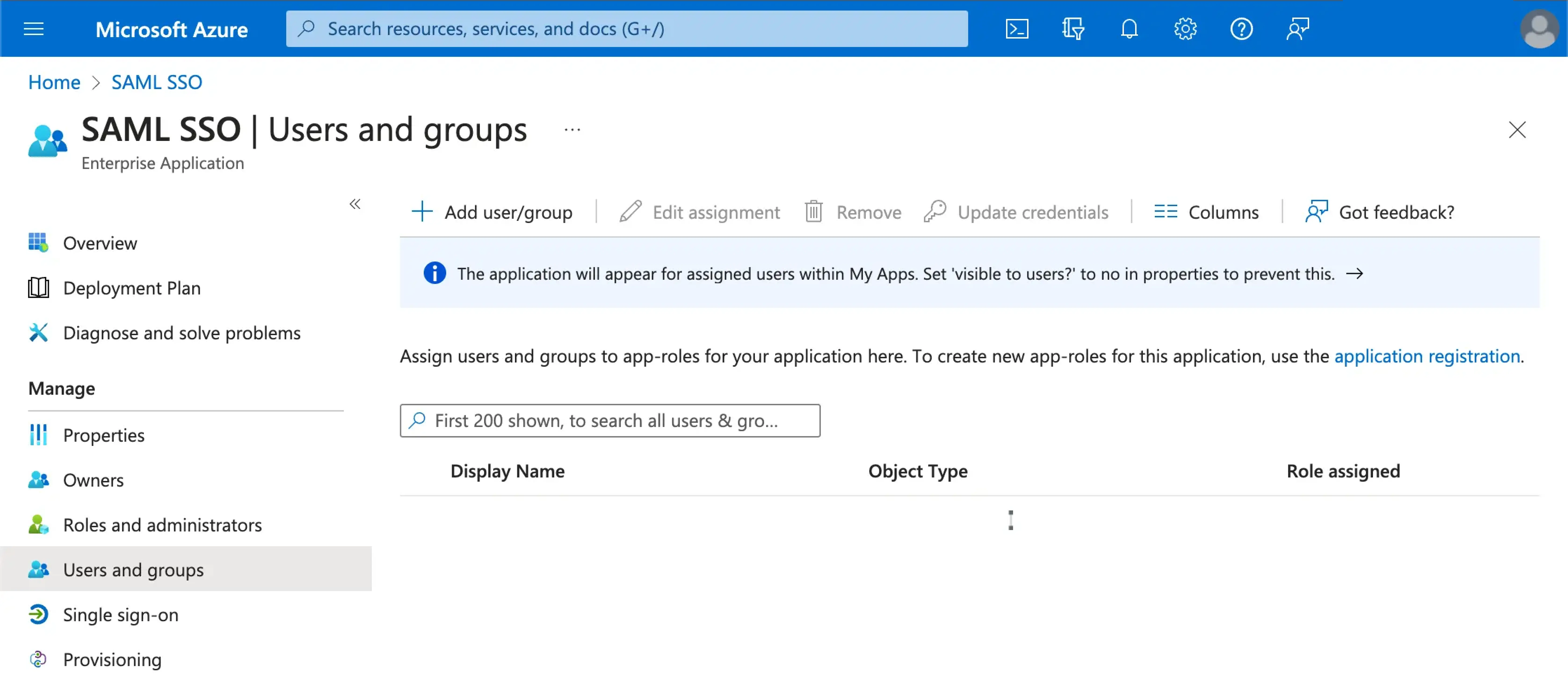
Step 5: Set email domains and enable the SSO connectorβ
Provide the email domains
of your organization at Logto's connector SSO experience
tab. This will enable the SSO connector as an authentication method for those users.
Users with email addresses in the specified domains will be redirected to use the SAML SSO connector as their only authentication method.
Please check Azure AD's official documentation for more details about the Azure AD SSO integration.
Save your configurationβ
Double check you have filled out necessary values in the Logto connector configuration area. Click "Save and Done" (or "Save changes") and the Microsoft Entra ID SAML enterprise SSO connector should be available now.
Enable Microsoft Entra ID SAML enterprise SSO connector in Sign-in Experienceβ
You donβt need to configure enterprise connectors individually, Logto simplifies SSO integration into your applications with just one click.
- Navigate to: Console > Sign-in experience > Sign-up and sign-in.
- Enable the "Enterprise SSO" toggle.
- Save changes.
Once enabled, a "Single Sign-On" button will appear on your sign-in page. Enterprise users with SSO-enabled email domains can access your services using their enterprise identity providers (IdPs).
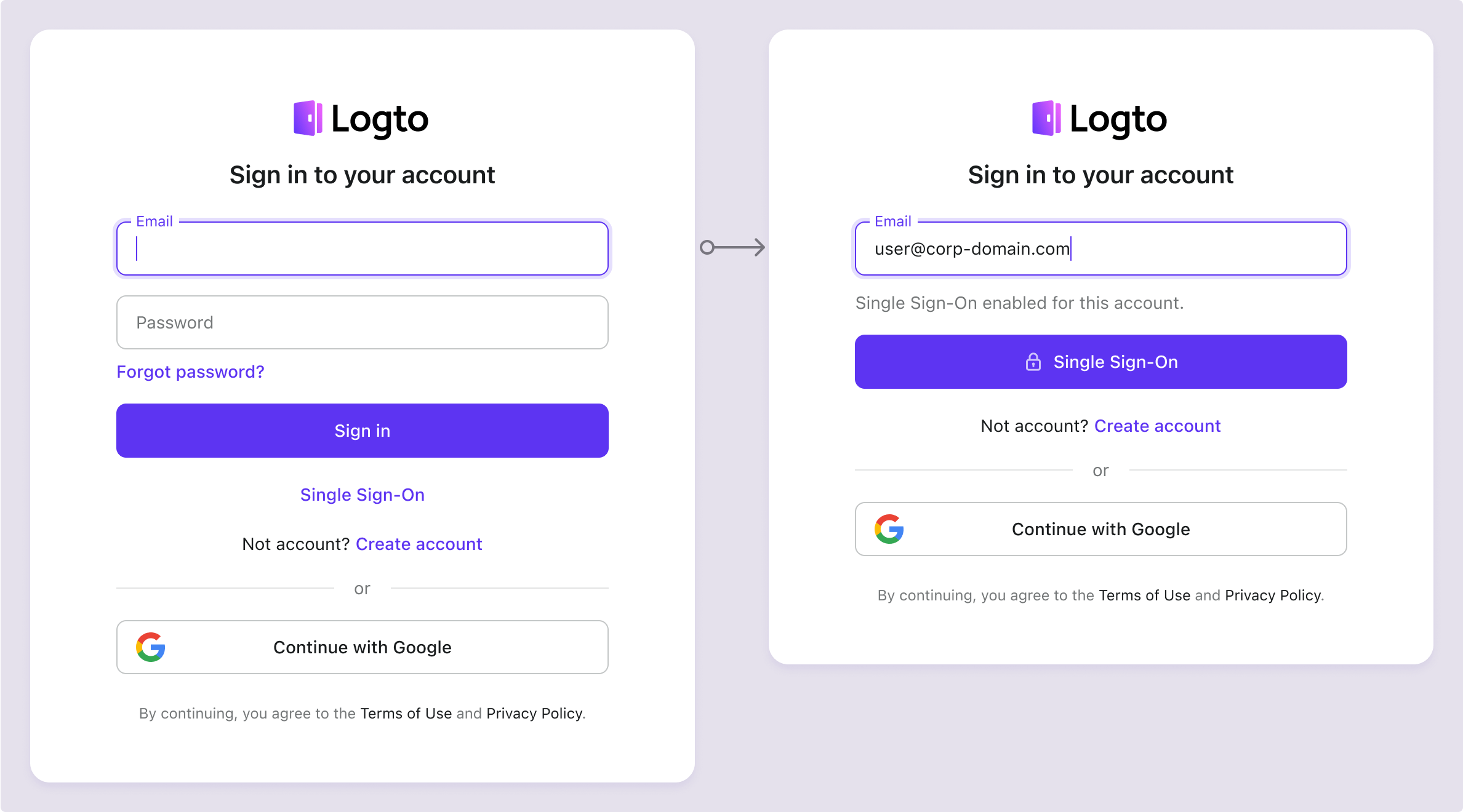
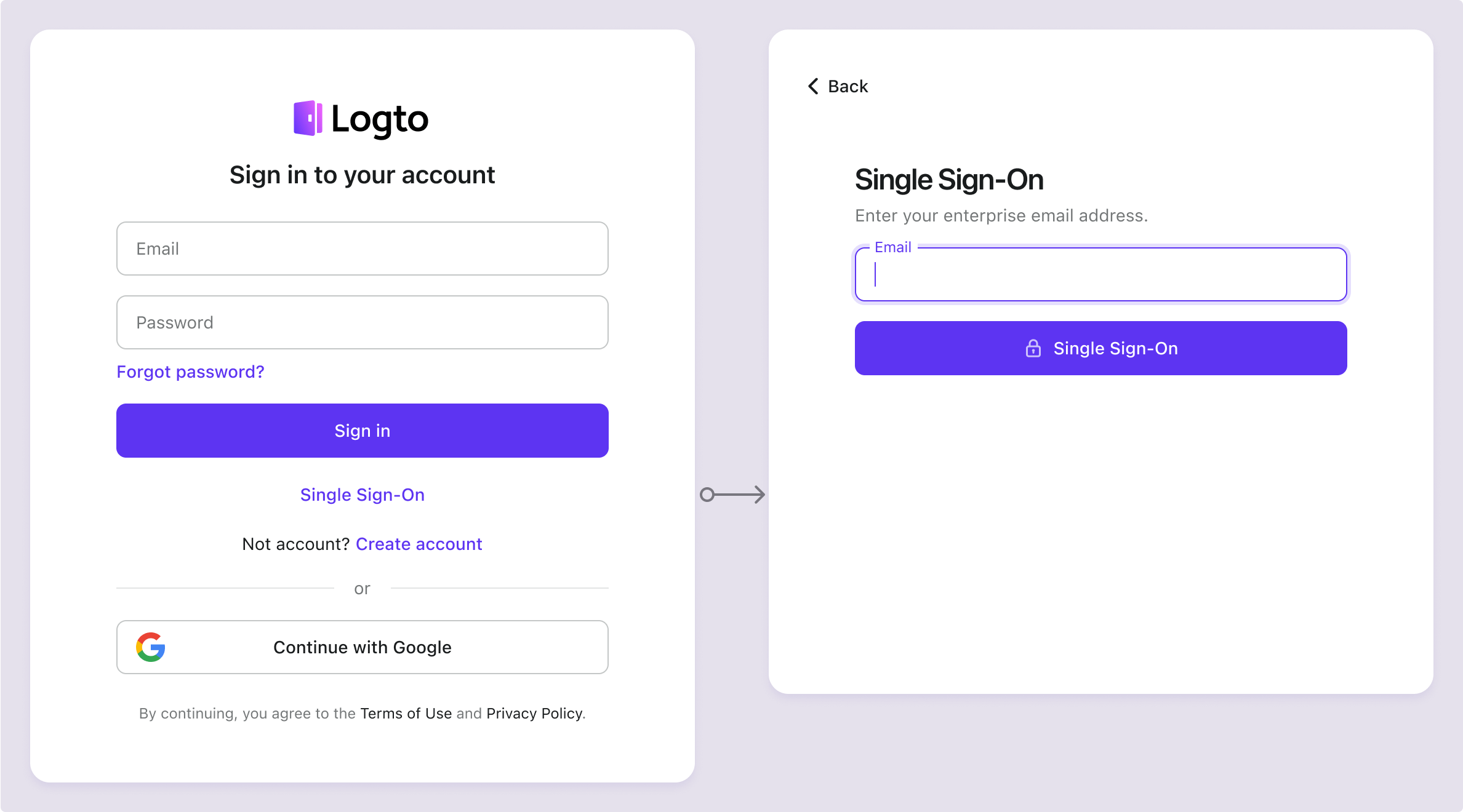
To learn more about the SSO user experience, including SP-initiated SSO and IdP-initiated SSO, refer to User flows: Enterprise SSO.
Testing and Validationβ
Return to your Expo (React Native) app. You should now be able to sign in with Microsoft Entra ID SAML enterprise SSO. Enjoy!
Further readingsβ
End-user flows: Logto provides a out-of-the-box authentication flows including MFA and enterprise SSO, along with powerful APIs for flexible implementation of account settings, security verification, and multi-tenant experience.
Authorization: Authorization defines the actions a user can do or resources they can access after being authenticated. Explore how to protect your API for native and single-page applications and implement Role-based Access Control (RBAC).
Organizations: Particularly effective in multi-tenant SaaS and B2B apps, the organization feature enable tenant creation, member management, organization-level RBAC, and just-in-time-provisioning.
Customer IAM series Our serial blog posts about Customer (or Consumer) Identity and Access Management, from 101 to advanced topics and beyond.