Logto is an Auth0 alternative designed for modern apps and SaaS products. It offers both Cloud and Open-source services to help you quickly launch your identity and management (IAM) system. Enjoy authentication, authorization, and multi-tenant management all in one.
We recommend starting with a free development tenant on Logto Cloud. This allows you to explore all the features easily.
In this article, we will go through the steps to quickly build the Twilio sign-in experience (user authentication) with Android (Kotlin / Java) and Logto.
Prerequisites
- A running Logto instance. Check out the introduction page to get started.
- Basic knowledge of Android (Kotlin / Java).
- A usable Twilio account.
Create an application in Logtoβ
Logto is based on OpenID Connect (OIDC) authentication and OAuth 2.0 authorization. It supports federated identity management across multiple applications, commonly called Single Sign-On (SSO).
To create your Native app application, simply follow these steps:
- Open the Logto Console. In the "Get started" section, click the "View all" link to open the application frameworks list. Alternatively, you can navigate to Logto Console > Applications, and click the "Create application" button.
- In the opening modal, click the "Native app" section or filter all the available "Native app" frameworks using the quick filter checkboxes on the left. Click the "Android" framework card to start creating your application.
- Enter the application name, e.g., "Bookstore," and click "Create application".
π Ta-da! You just created your first application in Logto. You'll see a congrats page which includes a detailed integration guide. Follow the guide to see what the experience will be in your application.
Integrate Android with Logtoβ
- The example is based on View system and View Model, but the concepts are the same when using Jetpack Compose.
- The example is written in Kotlin, but the concepts are the same for Java.
- Both Kotlin and Java sample projects are available on our SDK repository.
- The tutorial video is available on our YouTube channel.
Installationβ
The minimum supported Android API level of Logto Android SDK is level 24.
Before you install Logto Android SDK, ensure mavenCentral()
is added to your repository configuration in the Gradle project build file:
dependencyResolutionManagement {
repositories {
mavenCentral()
}
}
Add Logto Android SDK to your dependencies:
- Kotlin
- Groovy
dependencies {
implementation("io.logto.sdk:android:1.1.3")
}
dependencies {
implementation 'io.logto.sdk:android:1.1.3'
}
Since the SDK needs internet access, you need to add the following permission to your AndroidManifest.xml
file:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<!-- add internet permission -->
<uses-permission android:name="android.permission.INTERNET" />
<!-- other configurations... -->
</manifest>
Init LogtoClientβ
Create a LogtoViewModel.kt
and init LogtoClient
in this view model:
//...with other imports
import io.logto.sdk.android.LogtoClient
import io.logto.sdk.android.type.LogtoConfig
class LogtoViewModel(application: Application) : AndroidViewModel(application) {
private val logtoConfig = LogtoConfig(
endpoint = "<your-logto-endpoint>",
appId = "<your-app-id>",
scopes = null,
resources = null,
usingPersistStorage = true,
)
private val logtoClient = LogtoClient(logtoConfig, application)
companion object {
val Factory: ViewModelProvider.Factory = object : ViewModelProvider.Factory {
@Suppress("UNCHECKED_CAST")
override fun <T : ViewModel> create(
modelClass: Class<T>,
extras: CreationExtras
): T {
// Get the Application object from extras
val application = checkNotNull(extras[APPLICATION_KEY])
return LogtoViewModel(application) as T
}
}
}
}
then, create a LogtoViewModel
for your MainActivity.kt
:
//...with other imports
class MainActivity : AppCompatActivity() {
private val logtoViewModel: LogtoViewModel by viewModels { LogtoViewModel.Factory }
//...other codes
}
Configure redirect URIβ
Let's switch to the Application details page of Logto Console. Add a Redirect URI io.logto.android://io.logto.sample/callback
and click "Save changes".
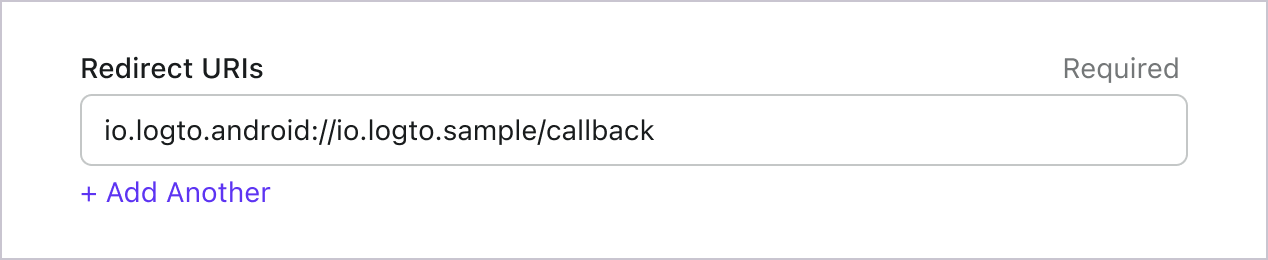
Implement sign-in and sign-outβ
Before calling logtoClient.signIn
, make sure you have correctly configured Redirect URI
in Admin Console.
You can use logtoClient.signIn
to sign in the user and logtoClient.signOut
to sign out the user.
For example, in an Android app:
//...with other imports
class LogtoViewModel(application: Application) : AndroidViewModel(application) {
// ...other codes
// Add a live data to observe the authentication status
private val _authenticated = MutableLiveData(logtoClient.isAuthenticated)
val authenticated: LiveData<Boolean>
get() = _authenticated
fun signIn(context: Activity) {
logtoClient.signIn(context, "io.logto.android://io.logto.sample/callback") { logtoException ->
logtoException?.let { println(it) }
// Update the live data
_authenticated.postValue(logtoClient.isAuthenticated)
}
}
fun signOut() {
logtoClient.signOut { logtoException ->
logtoException?.let { println(it) }
// Update the live data
_authenticated.postValue(logtoClient.isAuthenticated)
}
}
}
Then call the signIn
and signOut
methods in your activity:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
//...other codes
// Assume you have a button with id "sign_in_button" in your layout
val signInButton = findViewById<Button>(R.id.sign_in_button)
signInButton.setOnClickListener {
logtoViewModel.signIn(this)
}
// Assume you have a button with id "sign_out_button" in your layout
val signOutButton = findViewById<Button>(R.id.sign_out_button)
signOutButton.setOnClickListener {
if (logtoViewModel.authenticated) { // Check if the user is authenticated
logtoViewModel.signOut()
}
}
// Observe the authentication status to update the UI
logtoViewModel.authenticated.observe(this) { authenticated ->
if (authenticated) {
// The user is authenticated
signInButton.visibility = View.GONE
signOutButton.visibility = View.VISIBLE
} else {
// The user is not authenticated
signInButton.visibility = View.VISIBLE
signOutButton.visibility = View.GONE
}
}
}
}
Checkpoint: Test your applicationβ
Now, you can test your application:
- Run your application, you will see the sign-in button.
- Click the sign-in button, the SDK will init the sign-in process and redirect you to the Logto sign-in page.
- After you signed in, you will be redirected back to your application and see the sign-out button.
- Click the sign-out button to clear token storage and sign out.
Add Twilio connectorβ
SMS connector is a method used to send one-time passwords (OTPs) for authentication. It enables Phone number verification to support passwordless authentication, including SMS-based registration, sign-in, two-factor authentication (2FA), and account recovery. You can easily connect Twilio as your SMS provider. With the Logto SMS connector, you can set this up in just a few minutes.
To add a SMS connector, simply follow these steps:
- Navigate to Console > Connector > Email and SMS connectors.
- To add a new SMS connector, click the "Set up" button and select "Twilio".
- Review the README documentation for your selected provider.
- Complete the configuration fields in the "Parameter Configuration" section.
- Customize the SMS template using the JSON editor.
- Test your configuration by sending a verification code to your Phone number.
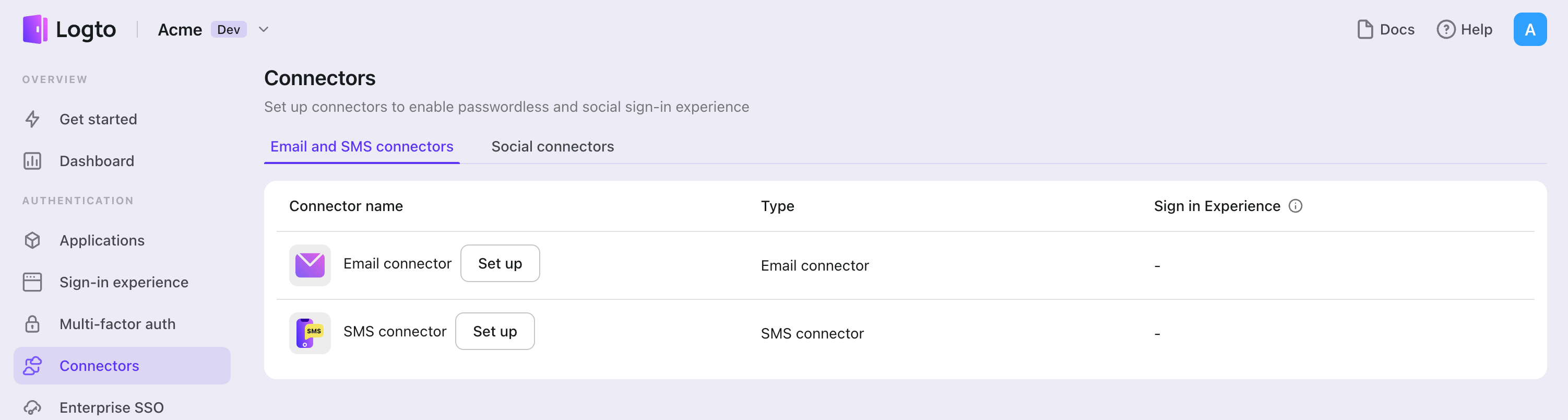
If you are following the in-place Connector guide, you can skip the next section.
Set up Twilio SMS connectorβ
Register Twilio accountβ
Create a new account on Twilio. (Jump to the next step if you already have one.)
Set up senders' phone numbersβ
Go to the Twilio console page and sign in with your Twilio account.
Purchase a phone number under "Phone Numbers" -> "Manage" -> "Buy a number".
Sometimes you may encounter the situation that SMS service is not supported in specific countries or areas. Pick a number from other regions to bypass.
Once we have a valid number claimed, nav to the "Messaging" -> "Services". Create a new Message Service by clicking on the button.
Give a friendly service name and choose Notify my users as our service purpose.
Following the next step, choose Phone Number
as Sender Type, and add the phone number we just claimed to this service as a sender.
Each phone number can only be linked with one messaging service.
Get account credentialsβ
We will need the API credentials to make the connector work. Let's begin from the Twilio console page.
Click on the "Account" menu in the top-right corner, then go to the "API keys & tokens" page to get your Account SID
and Auth token
.
Back to "Messaging" -> "Services" settings page starting from the sidebar, and find the Sid
of your service.
Compose the connector JSONβ
Fill out the accountSID, authToken and fromMessagingServiceSID fields with Account SID
, Auth token
and Sid
of the corresponding messaging service.
You can add multiple SMS connector templates for different cases. Here is an example of adding a single template:
- Fill out the
content
field with arbitrary string-typed contents. Do not forget to leave{{code}}
placeholder for random verification code. - Fill out the
usageType
field with eitherRegister
,SignIn
,ForgotPassword
,Generic
for different use cases. In order to enable full user flows, templates with usageTypeRegister
,SignIn
,ForgotPassword
andGeneric
are required.
Test Twilio SMS connectorβ
You can enter a phone number and click on "Send" to see whether the settings can work before "Save and Done".
That's it. Don't forget to Enable connector in sign-in experience.
Config typesβ
Name | Type |
---|---|
accountSID | string |
authToken | string |
fromMessagingServiceSID | string |
templates | Templates[] |
Template Properties | Type | Enum values |
---|---|---|
content | string | N/A |
usageType | enum string | 'Register' | 'SignIn' | 'ForgotPassword' | 'Generic' |
Save your configurationβ
Double check you have filled out necessary values in the Logto connector configuration area. Click "Save and Done" (or "Save changes") and the Twilio connector should be available now.
Enable Twilio connector in Sign-in Experienceβ
Once you create a connector successfully, you can enable phone number-based passwordless login and registration.
- Navigate to Console > Sign-in experience > Sign-up and sign-in.
- Set up sign-up methods (Optional):
- Select "Phone number" or "Email or phone number" as the sign-up identifier.
- "Verify at sign-up" is forced to be enabled. You can also enable "Create a password" on registration.
- Set up sign-in methods:
- Select Phone number as one of sign-in identifiers. You can provide multiple available identifiers (email, phone number, and username).
- Select "Verification code" and / or "Password" as the authentication factor.
- Click "Save changes" and test it in "Live preview".
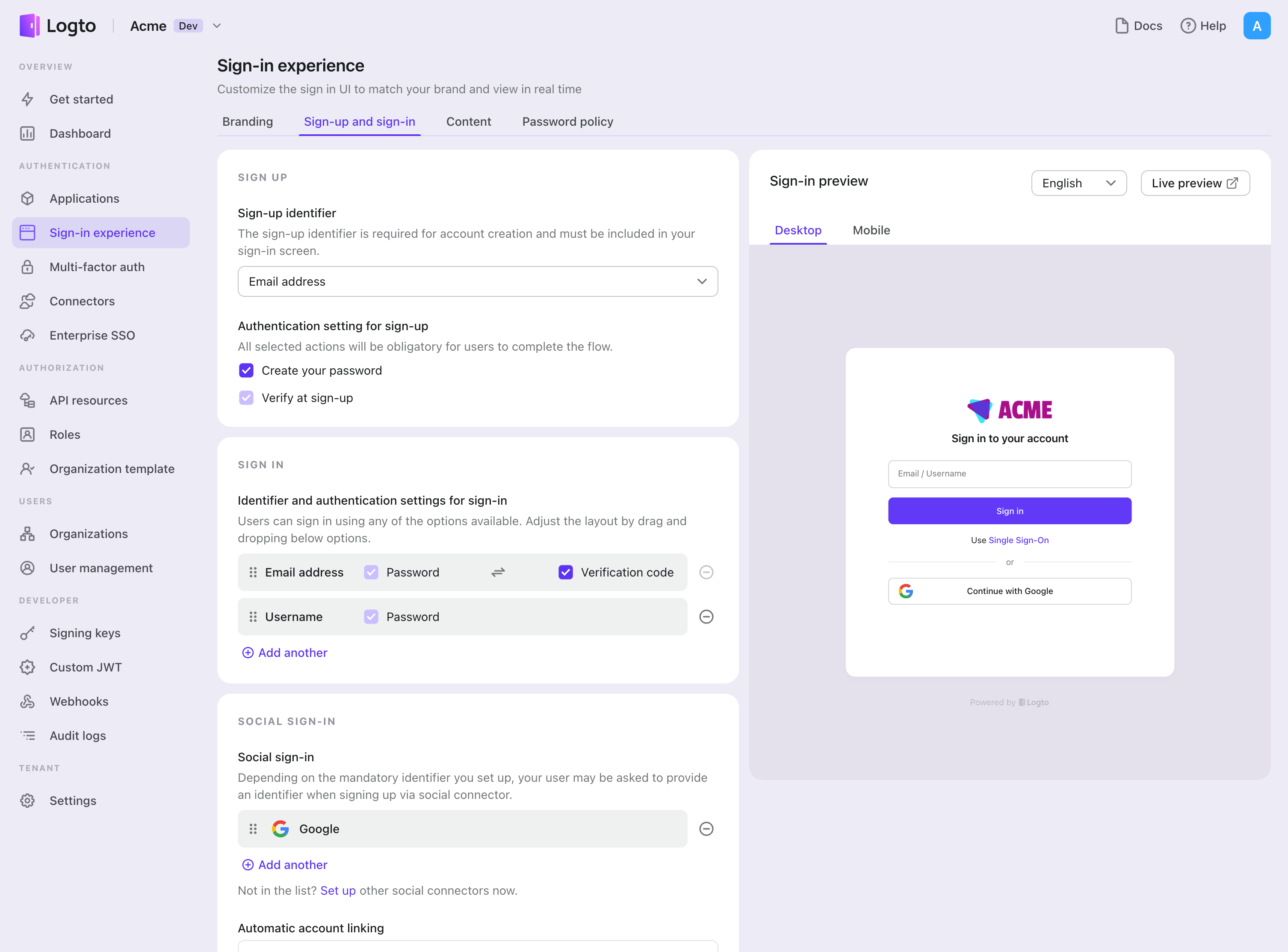
In addition to registration and login via OTPs, you can also have password recovery and -based security verification enabled, as well as linking Phone number to profile. See End-user flows for more details.
Testing and Validationβ
Return to your Android (Kotlin / Java) app. You should now be able to sign in with Twilio. Enjoy!
Further readingsβ
End-user flows: Logto provides a out-of-the-box authentication flows including MFA and enterprise SSO, along with powerful APIs for flexible implementation of account settings, security verification, and multi-tenant experience.
Authorization: Authorization defines the actions a user can do or resources they can access after being authenticated. Explore how to protect your API for native and single-page applications and implement Role-based Access Control (RBAC).
Organizations: Particularly effective in multi-tenant SaaS and B2B apps, the organization feature enable tenant creation, member management, organization-level RBAC, and just-in-time-provisioning.
Customer IAM series Our serial blog posts about Customer (or Consumer) Identity and Access Management, from 101 to advanced topics and beyond.